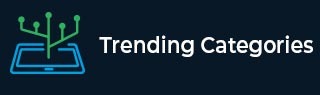
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Remove repeated digits in a given number using C++
In this article, we are given a number n, and we need to remove repeated digits in a given number.
Input: x = 12224 Output: 124 Input: x = 124422 Output: 1242 Input: x = 11332 Output: 132
In the given problem, we will go through all the digits and remove the repeating digits.
Approach to find The Solution
In the given approach, we will go through all the digits of n from right to left now. We go through digits of n by taking mod of n with 10 and then dividing n with 10. Now our current digit is n mod 10. We check it with the previous digit. If the digits are equal, we traverse n now. If they are not similar, we add this digit to our new number, change the previous digit to current, and continue the loop.
Example
#include <bits/stdc++.h> #define MOD 1000000007 using namespace std; int main() { int n = 1222333232; // given n int new_n = 0; // new number int po = 1; // will b multiple of ten for new digits int prev = -1; // previous digit int curr; // current digit while(n) { curr = n % 10; if(prev != curr) { // if a digit is not repeated then we go in this block new_n = new_n + (curr * po); // we add a new digit to new_n po *= 10; prev = curr; } n /= 10; } cout << new_n << "\n"; return 0; }
Output
123232
Explanation of the above code
In the above approach, we are simply traversing through the digits of n now when our previous digit and current digit don’t match, we add such digit to our new number, and as our digit is added, we also increase po, which is being used for positions of our digits now if our current and previous digits match-we don’t run this block and continue the loop till our n becomes 0.
Conclusion
In this article, we solve a problem to Remove repeated digits in a given number. We also learned the C++ program for this problem and the complete approach ( Normal) by which we solved this problem. We can write the same program in other languages such as C, java, python, and other languages. We hope you find this article helpful.