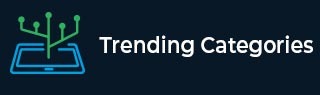
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Reflect Array class in Java
The Array class of the java.lang.reflect package provides static methods to create and access Java arrays dynamically. Array permits widening conversions to occur during a get or set operation but throws an IllegalArgumentException if a narrowing conversion would occur.
This class provides the newInstance() method, getter method, and setter methods. The newInstance() method accepts an object of the class named Class representing the required component and an integer representing the length of the array, creates and returns an array with the given specifications.
Example
import java.lang.reflect.Array; import java.util.Arrays; public class Reflection_ArrayExample { public static void main(String args[]){ Integer [] intArray = (Integer[]) Array.newInstance(Integer.class, 5); intArray[0] = 2001; intArray[1] = 12447; intArray[2] = 6358; intArray[3] = 902; intArray[4] = 6654; System.out.println(Arrays.toString(intArray)); } }
Output
[2001, 12447, 6358, 902, 6654]
The getter methods of this class getLong(), getInteger(), getLong(), etc. accepts an array in the form of an object and an integer representing an index and returns the element of the given array in the specified index.
The setter methods of this class setLong(), setInteger(), setLong() etc. accepts an array in the form of an object, an integer representing the index and a value of the respective datatype and sets the given value at the specified index.
Example
import java.lang.reflect.Array; import java.util.Arrays; public class Reflection_ArrayExample { public static void main(String args[]){ int [] intArray = (int[]) Array.newInstance(int.class, 5); intArray[0] = 2001; intArray[1] = 12447; intArray[2] = 6358; intArray[3] = 902; intArray[4] = 6654; Array.setInt(intArray, 1, 1111); Array.setInt(intArray, 3, 3333); Array.setInt(intArray, 4, 4444); System.out.println(Arrays.toString(intArray)); System.out.println(Array.getInt(intArray, 1)); System.out.println(Array.getInt(intArray, 3)); System.out.println(Array.getInt(intArray, 4)); } }
Output
[2001, 1111, 6358, 3333, 4444] 1111 3333 4444