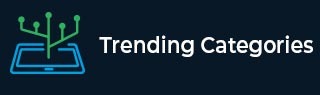
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Reduce the fraction to its lowest form in C++
Given two integers Num1 and Num2 as input. The integers can be represented as fraction Num1/Num2. The goal is to reduce this fraction to its lowest form.
Using GCD to find highest denominator
We will calculate the greatest common divisor of both numbers.
Divide both numbers by that gcd
Set both variables as quotient after division.
Lowest fraction will be Num1/Num2.
Examples
Input − Num1=22 Num2=10
Output − Num1 = 11 Num2 = 5
Lowest Fraction : 11/5
Explanation− GCD of 22 and 10 is 2.
22/2=11 and 10/2=5
Lowest fraction is 11/5
Input− Num1=36 Num2=40
Output− Num1 = 9 Num2 = 10
Lowest Fraction : 9/10
Explanation − GCD of 36 and 40 is 4.
40/4=10 and 36/4=9
Lowest fraction is 9/10
Approach used in the below program is as follows
In this approach we will first calculate the GCD of input numbers using a recursive approach. Divide both numbers by GCD and obtain quotients. These quotients will be part of the lowest fraction.
Take the input variables Num1 and Num2.
Function findGCD(int a, int b) takes num1 and num2 and returns the gcd of both.
If b is 0 return a else return findGCD(b,a%b).
Function lowestFraction(int num1, int num2) takes both numbers as input and prints the lowest fraction.
Take variable denom for gcd.
Set num1=num1/denom and num2=num2/denom.
Print num1 and num2.
Print the lowest fraction as num1/num2.
Example
#include <bits/stdc++.h> using namespace std; int findGCD(int a, int b) { if (b == 0) return a; return findGCD(b, a % b); } void lowestFraction(int num1, int num2){ int denom; denom = findGCD(num1,num2); num1/=denom; num2/=denom; cout<< "Num1 = " << num1<<endl; cout<< "Num2 = " << num2<<endl; cout<< "Lowest Fraction : "<<num1<<"/"<<num2; } int main(){ int Num1 = 14; int Num2 = 8; lowestFraction(Num1,Num2); return 0; }
Output
If we run the above code it will generate the following Output
Num1 = 7 Num2 = 4 Lowest Fraction : 7/4