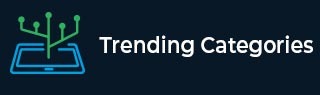
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Recursively print all sentences that can be formed from list of word lists in C++
Given a list of words. The goal is to create all possible sentences that can be formed by taking words from the list using a recursive approach. You can only take one word at a time from both the lists.
Let us see various input output scenarios for this
Input −
sentence[row][col] = {{"I", "You"}, {"Do", "do not like"}, {"walking", "eating"}}
Output −
I Do walking I Do eating I like walking I like eating You Do walking You Do eating You like walking You like eating
Explanation − Taking one word from each list in sentence[0-2] gives above sentences.
Input −
sentence[row][col] = {{"work", "live"},{"easy", "happily"}}
Output −
work easy work happily live easy live happily
Explanation − Taking one word from each list in sentence[0-1] gives above sentences.
Declare a 2-D array of string type as sentence[row][col]. Pass the data to the function as Recursive_Print(sentence).
Inside the function as Recursive_Print(sentence)
Create an array of type string as arr[row].
Start loop FOR from i to 0 till i is less than col. Inside the loop, check IF sentence[0][i] is not empty then make a call to the function as Recursion(sentence, 0, i, arr).
Inside the function as Recursion(string sentence[row][col], int temp_1, int temp_2, string arr[row])
Set arr[temp_1] to sentence[temp_1][temp_2].
Check IF temp_1 is row - 1 then start loop FOR from i to 0 till i less than row. Inside the loop, print arr[i].
Start loop FOR from i to 0 till i is less than col. Inside the loop, check IF sentence[temp_1+1][i] is not equal to space then make a recursive call to the function as Recursion(sentence, temp_1+1, i, arr).
Print the result.
Approach used in the below program is as follows
Example
#include<bits/stdc++.h> #define row 3 #define col 3 using namespace std; void Recursion(string sentence[row][col], int temp_1, int temp_2, string arr[row]){ arr[temp_1] = sentence[temp_1][temp_2]; if(temp_1 == row - 1){ for(int i=0; i < row; i++){ cout << arr[i] << " "; } cout << endl; return; } for(int i=0; i < col; i++){ if(sentence[temp_1+1][i] != ""){ Recursion(sentence, temp_1+1, i, arr); } } } void Recursive_Print(string sentence[row][col]){ string arr[row]; for(int i=0; i < col; i++){ if(sentence[0][i] != ""){ Recursion(sentence, 0, i, arr); } } } int main(){ string sentence[row][col] = {{"Ajay", "sanjay"},{"Like", "is"},{"Reading", "eating"}}; Recursive_Print(sentence); return 0; }
Output
If we run the above code it will generate the following Output
Ajay Like Reading Ajay Like eating Ajay is Reading Ajay is eating sanjay Like Reading sanjay Like eating sanjay is Reading sanjay is eating