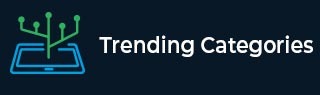
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Recursively loop through an array and return number of items with JavaScript?
We have to write a function, say searchRecursively() that takes in an array and a search query and returns the count of that search query in the nested array.
For example, if the array is given by −
const names = ["rakesh", ["kalicharan", "krishna", "rakesh", "james", ["michael", "nathan", "rakesh", "george"]]];
Then −
searchRecursively(names, ‘’rakesh’);
Should return 3 because it makes a total of 3 appearances in the array. Therefore, let’s write the code for this recursive function −
Example
const names = ["rakesh", ["kalicharan", "krishna", "rakesh", "james", ["michael", "nathan", "rakesh", "george"]]]; const searchRecursively = (arr, query, count = 0, len = 0) => { if(len < arr.length){ if(Array.isArray(arr[len])){ return searchRecursively(arr[len], query, count, 0); }; if(arr[len] === query){ return searchRecursively(arr, query, ++count, ++len); }; return searchRecursively(arr, query, count, ++len); }; return count; }; console.log(searchRecursively(names, "rakesh"));
Output
The output in the console will be −
3
Advertisements