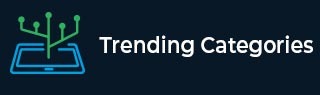
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Rearranging cards into groups in JavaScript
Problem
We are required to write a JavaScript function that takes in an array of numbers, arr, as the first argument and a number, num, as the second argument.
The numbers in the array are in the range [1, 13], limits inclusive, representing the 1-based index of playing cards.
Our function should determine whether there exists a way to rearrange the cards into groups so that each group is size num, and consists of num consecutive cards.
For example, if the input to the function is
Input
const arr = [1, 4, 3, 2]; const num = 2;
Output
const output = 2;
Output Explanation
Because the cards can be rearranged as [1, 2], [3, 4]
Example
Following is the code −
const arr = [1, 4, 3, 2]; const num = 2; const canRearrange = (arr = [], num = 1) => { const find = (map, n, num) => { let j = 0 while(j < num) { if(!map[n + j]) return false else map[n + j] -= 1 j++ } return true } let map = {} arr.sort(function(a, b) {return a - b}) for(let n of arr) { map[n] = map[n] ? map[n] + 1 : 1 } for(let n of arr) { if(map[n] === 0 || find(map, n, num)) continue else return false } return true }; console.log(canRearrange(arr, num));
Output
true
Advertisements