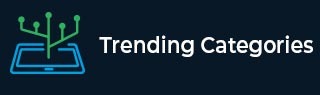
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Rearrange String k Distance Apart in C++
Suppose we have a non-empty string s and an integer k; we have to rearrange the string such that the same characters are at least distance k from each other. Given strings are in lowercase letters. If there is no way to rearrange the strings, then we will an empty string.
So, if the input is like s = "aabbcc", k = 3, then the output will be "abcabc" this is because same letters are at least distance 3 from each other.
To solve this, we will follow these steps −
ret := an empty string
Define one map m
n := size of s
for initialize i := 0, when i < n, update (increase i by 1), do −
(increase m[s[i]] by 1)
Define one priority queue pq
for each key-value pair it in m, do −
temp := a pair with key and value of it
insert temp into pq
(increase it by 1)
Define one deque dq
while (not pq is empty), do −
curr := top of pq
delete element from pq
ret := ret + curr.first
(decrease curr.second by 1)
insert curr at the end of dq
if size of dq >= k, then −
curr := first element of dq
delete front element from dq
if curr.second > 0, then −
insert curr into pq
while (not dq is empty and first element of dq is same as 0), do −
delete front element from dq
return (if dq is empty, then ret, otherwise blank string)
Example
Let us see the following implementation to get better understanding −
#include <bits/stdc++.h> using namespace std; struct Comparator { bool operator()(pair<char, int> a, pair<char, int> b) { return !(a.second > b.second); } }; class Solution { public: string rearrangeString(string s, int k) { string ret = ""; unordered_map<char, int> m; int n = s.size(); for (int i = 0; i < n; i++) { m[s[i]]++; } unordered_map<char, int>::iterator it = m.begin(); priority_queue<pair<char, int<, vector<pair<char, int>>, Comparator> pq; while (it != m.end()) { pair<char, int> temp = {it->first, it->second}; pq.push(temp); it++; } deque<pair<char, int>> dq; while (!pq.empty()) { pair<char, int> curr = pq.top(); pq.pop(); ret += curr.first; curr.second--; dq.push_back(curr); // cout << ret << " " << dq.size() << endl; if (dq.size() >= k) { curr = dq.front(); dq.pop_front(); if (curr.second > 0) pq.push(curr); } } while (!dq.empty() && dq.front().second == 0) dq.pop_front(); return dq.empty() ? ret : ""; } }; main() { Solution ob; cout << (ob.rearrangeString("aabbcc", 3)); }
Input
"aabbcc", 3
Output
bacbac