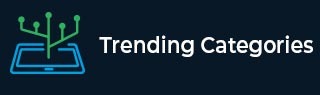
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Reading and Writing to text files in Python
Like other languages, Python provides some inbuilt functions for reading, writing, or accessing files. Python can handle mainly two types of files. The normal text file and the binary files.
For the text files, each lines are terminated with a special character '\n' (It is known as EOL or End Of Line). For the Binary file, there is no line ending character. It saves the data after converting the content into bit stream.
In this section we will discuss about the text files.
File Accessing Modes
Sr.No | Modes & Description |
---|---|
1 | r It is Read Only mode. It opens the text file for reading. When the file is not present, it raises I/O Error. |
2 | r+ This mode for Reading and Writing. When the file is not present, it will raise I/O Error. |
3 | w It is for write only jobs. When file is not present, it will create a file first, then start writing, when the file is present, it will remove the contents of that file, and start writing from beginning. |
4 | w+ It is Write and Read mode. When file is not present, it can create the file, or when the file is present, the data will be overwritten. |
5 | a This is append mode. So it writes data at the end of a file. |
6 | a+ Append and Read mode. It can append data as well as read the data. |
Now see how a file can be written using writelines() and write() method.
Example code
#Create an empty file and write some lines line1 = 'This is first line. \n' lines = ['This is another line to store into file.\n', 'The Third Line for the file.\n', 'Another line... !@#$%^&*()_+.\n', 'End Line'] #open the file as write mode my_file = open('file_read_write.txt', 'w') my_file.write(line1) my_file.writelines(lines) #Write multiple lines my_file.close() print('Writing Complete')
Output
Writing Complete
After writing the lines, we are appending some lines into the file.
Example code
#program to append some lines line1 = '\n\nThis is a new line. This line will be appended. \n' #open the file as append mode my_file = open('file_read_write.txt', 'a') my_file.write(line1) my_file.close() print('Appending Done')
Output
Appending Done
At last, we will see how to read the file content from the read() and readline() method. We can provide some integer number 'n' to get first 'n' characters.
Example code
#program to read from file #open the file as read mode my_file = open('file_read_write.txt', 'r') print('Show the full content:') print(my_file.read()) #Show first two lines my_file.seek(0) print('First two lines:') print(my_file.readline(), end = '') print(my_file.readline(), end = '') #Show upto 25 characters my_file.seek(0) print('\n\nFirst 25 characters:') print(my_file.read(25), end = '') my_file.close()
Output
Show the full content: This is first line. This is another line to store into file. The Third Line for the file. Another line... !@#$%^&*()_+. End Line This is a new line. This line will be appended. First two lines: This is first line. This is another line to store into file. First 25 characters: This is first line. This