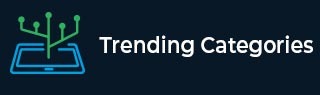
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Read and Write Input in Dart Programming
Dart provides us with a standard library named 'io' which contains different classes and in turn, these classes contains different methods that we can use to read or write input from the terminal.
We import the library in our program by making use of the import command.
Example
Consider the example shown below −
Import 'dart:io';
Writing something to the terminal
We can write something to the terminal by making use of the standard out class (stdout) that is available to us in the 'dart:io' library.
Example
Consider the example shown below −
import 'dart:io'; void main(List<String> arguments) { stdout.write('What is your name?\r
'); }
Output
What is your name?
Note − It should be noted that we run a dart file by running the command: dart run <nameofyourdartfile>
Reading user input
We can read the input provided by the use with the help of the standard input class (stdin) that is available to us in the 'dart:io' library.
Example
Consider the example shown below −
import 'dart:io'; void main(List<String> arguments){ stdout.write("What is your name?\r
"); var name = stdin.readLineSync(); print(name); }
In the above code example, we are trying to write the string "What is your name?" to the console, and then later we are asking for user input and storing that input in the name variable and then finally we are printing that variable.
Output
What is your name? mukul mukul
It should be noted that there might be a case when the user doesn't enter anything and to handle that case we need to check if the user entered nothing, we can do that by making use of the null aware operators that dart provides us.
Example
Consider the example shown below −
import 'dart:io'; void main(List<String> arguments){ stdout.write("What is your name?\r
"); var name; name = name ?? stdin.readLineSync(); name.isEmpty ? stdout.write('Enter a name
') : stdout.write('Welcome ${name}
'); }
In the above code example, if we encounter a case where the user entered nothing we can simply return a standard message to the terminal, saying 'Enter a name'.
Output
What is your name? Enter a name