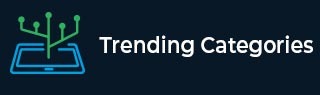
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
ReactJS – useContext hook
In this article, we are going to see how to access the data without passing it through every parent component in the React Lifecycle.
This hook is the better replacement of the Context API of the Class-based component which is used to set the global data and this data can now be accessed in any of the children components without the need to pass it through every parent Component.
Syntax
const authContext = useContext(initialValue);
The useContext accepts the value provided by React.createContext and then re-render the component whenever its value changes but you can still optimize its performance by using memoization.
Example
In this example, we are going to build an authentication React application that logs in or logs out users in the children component and updates the state accordingly.
We will build an App Component which has two LogIn and LogOut Components which access the state provided by the Context Provider and updates it accordingly.
AuthContext.js
import React from 'react'; const authContext = React.createContext({ auth: null, login: () => {}, logout: () => {}, }); export default authContext;
App.jsx
import React, { useState } from 'react'; import LogIn from './Login'; import LogOut from './Logout'; import AuthContext from './AuthContext'; const App = () => { const [auth, setAuth] = useState(false); const login = () => { setAuth(true); }; const logout = () => { setAuth(false); }; return ( <React.Fragment> <AuthContext.Provider value={{ auth: auth, login: login, logout: logout }} > <p>{auth ? 'Hi! You are Logged In' : 'Oope! Kindly Login'}</p> <LogIn /> <LogOut /> </AuthContext.Provider> </React.Fragment> ); }; export default App;
Login.js
import React, { useContext } from 'react'; import AuthContext from './AuthContext'; const Login = () => { const auth = useContext(AuthContext); return ( <> <button onClick={auth.login}>Login</button> </> ); }; export default Login;
Logout.js
import React, { useContext } from 'react'; import AuthContext from './AuthContext'; const Logout = () => { const auth = useContext(AuthContext); return ( <> <button onClick={auth.logout}>Click To Logout</button> </> ); }; export default Logout;
Output
This will produce the following result.