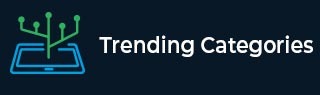
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
PyTorch – How to compute the pseudoinverse of a matrix?
To compute the pseudoinverse of a square matrix, we could apply torch.linalg.pinv() method. It returns a new tensor with pseudoinverse of the given matrix. It accepts a matrix, a batch of matrices and also batches of matrices. A matrix is a 2D torch Tensor. It supports input of float, double, cfloat, and cdouble data types.
Syntax
torch.linalg.pinv(M)
Where M is a matrix or batches of matrices.
Steps
We could use the following steps to compute the pseudoinverse of a matrix −
Import the required library. In all the following examples, the required Python library is torch. Make sure you have already installed it.
import torch
Define a matrix. Here, we define a matrix (2D tensor of size 3x4.
M = torch.randn(3,4)
Compute the pseudoinverse matrix using torch.linalg.pinv(M). M is a matrix or batch/es of matrices. Optionally assign this value to a new variable.
Mpinv = torch.linalg.pinv(M)
Print the above computed pseudoinverse matrix
print("Norm:", Mpinv)
Example 1
In this program, we compute the pseudoinverse matrix of a given input matrix.
# Python program to compute the pseudoinverse of a matrix # import required library import torch # define a matrix of size 3x4 M = torch.randn(3,4) print("Matrix M:
", M) print("Matrix size:", M.size()) # compute the inverse of above defined matrix Mpinv = torch.linalg.pinv(M) print("Pseudo inverse Matrix:
", Mpinv) print("Pseudo inverse Matrix size:", Mpinv.size())
Output
It will produce the following output −
Matrix M: tensor([[ 1.1350, -1.0521, -0.6431, -0.1302], [-0.5745, 1.2299, 0.9296, 1.6188], [ 0.6129, -1.0834, -0.0711, 0.2382]]) Matrix size: torch.Size([3, 4]) Pseudo inverse Matrix: tensor([[ 1.1440, 0.3123, -0.6687], [ 0.7733, 0.2948, -1.3105], [-0.8647, -0.0376, 0.9169], [ 0.3150, 0.5262, 0.2319]]) Pseudo inverse Matrix size: torch.Size([4, 3])
Example 2
In this program, we compute the pseudoinverse matrix of a given input complex matrix.
# Python program to compute the # pseudo inverse of a complex matrix # import required library import torch # define a 3x2 matrix of random complex numbers M = torch.randn(3,2, dtype = torch.cfloat) print("Matrix M:
", M) print("Matrix size:", M.size()) # compute the inverse of above defined matrix Minv = torch.linalg.pinv(M) print("Pseudo inverse Matrix:
", Minv) print("Pseudo inverse Matrix size:", Minv.size())
Output
It will produce the following output −
Matrix M: tensor([[ 0.5273-0.7986j, 0.7881+0.0765j], [-0.6390-0.3126j, -0.1926+0.0727j], [-0.7445-0.2163j, 0.0649+0.1611j]]) Matrix size: torch.Size([3, 2]) Pseudo inverse Matrix: tensor([[ 0.0384+0.2124j, -0.3826+0.3125j, -0.5792+0.1700j], [ 0.9675-0.1972j, -0.2763-0.5200j, 0.2895-0.6992j]]) Pseudo inverse Matrix size: torch.Size([2, 3])
Example 3
In this program, we compute the pseudoinverse of a batch of three matrices.
# Python program to compute the # pseudo inverse of batch of matrices # import the required library import torch # define a batch of three 3x2 matrices B = torch.randn(3,3,2) print("Batch of Matrices :
", B) print(B.size()) # compute the inverse of above defined batch of matrices Binv = torch.linalg.pinv(B) print("Pseudo inverse Matrices:
", Binv) print(Binv.size())
Output
It will produce the following output −
Batch of Matrices : tensor([[[-0.1761, -1.4982], [ 0.7792, -0.0071], [-0.6187, -0.2396]], [[-1.1825, 1.2347], [ 0.0127, 1.0387], [ 0.0319, 0.5046]], [[-1.2648, -0.7298], [-1.8663, -1.1158], [-0.0148, 0.5049]]]) torch.Size([3, 3, 2]) Pseudo inverse Matrices: tensor([[[ 0.0932, 0.8222, -0.6073], [-0.6673, -0.1483, 0.0032]], [[-0.8261, 0.7798, 0.4164], [ 0.0185, 0.7538, 0.3850]], [[-0.2850, -0.3336, -1.1492], [ 0.0613, -0.0569, 1.9435]]]) torch.Size([3, 2, 3])