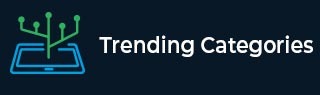
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python3 Program for Longest subsequence of a number having same left and right rotation
In this problem, we will find the length of the longest subsequence of the given string such that it has the same left and right rotation.
We can solve the problem by finding all subsequences of the given string and checking whether particular subsequences have the same left and right rotation. The other approach uses the observation that string can have only the same left and right rotation if it contains a single character or alternate character and the left is even.
Problem statement – We have given an alpha string containing only numeric digits. We need to find the size of the longest subsequence of the string, which has the same left and right rotations.
Sample examples
Input
alpha = "700980503"
Output
4
Explanation – The longest subsequence having the same left and right rotation is ‘0000’.
Input
alpha = ‘19199019’
Output
6
Explanation – The resultant subsequence is ‘191919’.
Input
alpha = ‘13141115611171’
Output
9
Explanation – The longest subsequence having the same left and right rotation is ‘111111111’.
Approach 1
In this approach, we will find all subsequences of the given string. After that, we will use the Python array slicing to check whether the subsequence has the same left and right rotation.
Algorithm
Step 1 – Initialize the ‘maxLen’ with -1 to store the maximum length of the subsequence.
Step 2 – Call the getSubs() function to get all the subsequences of the given string.
Step 2.1 – In the getSubs() function, initialize the ‘allSubs’ array with a single empty string element.
Step 2.2 – Start traversing the string, and create the copy of the array in the ‘temp’ variable.
Step 2.3 – Traverse the ‘temp’ array and append the ‘ch’ character to the subsequence of the temp array. After that, insert a new subsequence to the ‘allSubs’ array.
Step 2.4 – Return the ‘allSubs’ array.
Step 3 – After getting all subsequences, traverse the array of subsequences.
Step 4 – Slice the subsequence using the subseq[1:] + subseq[:1] to get the left rotation. Also, slice the subsequence using the subseq[str_len - 2:] + subseq[:str_len - 2] to get the right rotation.
Step 5 – If left and right rotation is the same, update the maxLen value if it is less than the subsequence’s length.
Step 6 – Return the ‘maxLen’ variable’s value.
Example
def getSubs(alpha): allSubs = [''] for ch in alpha: # Create a copy of the array containing all subsequences temp = allSubs[:] # Append character to each subsequence of the array to generate new subsequences for subseq in temp: allSubs.append(subseq + ch) return allSubs def maxSubSeqLen(alpha): maxLen = -1 str_len = len(alpha) allSubs = getSubs(alpha) # Traverse all subsequences for subseq in allSubs: # check if the subsequence has the same left and right rotation if subseq[1:] + subseq[:1] == subseq[str_len - 2:] + subseq[:str_len - 2]: maxLen = max(maxLen, len(subseq)) # Return the answer return maxLen alpha = "1919019" print("The maximum length of subsequence having the same left and right rotations is - ") print(maxSubSeqLen(alpha))
Output
The maximum length of subsequence having the same left and right rotations is - 6
Time complexity – O(2N), as we find all subsequences of the given string.
Space complexity – O(2N), as we store all subsequences of the given string.
Approach 2
In this approach, we will use observation based on the input and output of the problem. The string can only have the left and right rotation the same if the string contains all the same characters or is of even length and contains alternate characters.
So, we will find the longest subsequences according to both conditions.
Algorithm
Step 1 – Initialize the ‘maxLen’ variable with -1.
Step 2 – Use the loop to traverse from 0 to 9. Also, use another nested loop to traverse 0 to 9. Here, we make pair of digits such as 00, 01, 02, … 01, 12, 13, 14,… 97, 98, 99, etc. So, we will find the pair of digits in the given string and take the maximum length of the subsequence.
Step 3 –Initialize the ‘currMax’ and ‘isSecond’ variables with 0 to store the total number of existing pairs of numbers in the given string and to keep track of finding the first or second character of the pair in the string to create alternate subsequence.
Step 4 - Start traversing the string. If the ‘isSecond’ is equal to 0, and the character is equal to ‘p’, change ‘isSecond’ to 1 and increment the ‘currMax’ value by 1.
Step 5 - If the ‘isSecond’ is equal to 1, and the character is equal to ‘q’, change ‘isSecond’ to 0 and increment the ‘currMax’ value by 1.
Step 6 – If p and q are not the same, and ‘currMax’ is odd, decrease its value by 1.
Step 7 – Update the value of the ‘maxLen’ if it is less than the ‘currMax’ variable’s value.
Step 8 – Return the ‘maxLen’ value.
Example
def maxSubSeqLen(alpha): # Length of the string str_len = len(alpha) maxLen = -1 # Travere the string for p in range(10): for q in range(10): currMax, isSecond = 0, 0 # Find an alternate combination of p and q for k in range(str_len): # Finding the p if (isSecond == 0 and ord(alpha[k]) - ord('0') == p): isSecond = 1 # Increase the current value by 1 currMax += 1 # Finding q elif (isSecond == 1 and ord(alpha[k]) - ord('0') == q): isSecond = 0 # Increase the current value by 1 currMax += 1 # For odd length of resultant subsequence. if p != q and currMax % 2 == 1: # Decrease the value of currMax by 1 currMax -= 1 # Get maximum length maxLen = max(currMax, maxLen) # Return the answer return maxLen # Driver code alpha = "700980503" print("The maximum length of subsequence having the same left and right rotations is - ") print(maxSubSeqLen(alpha))
Output
The maximum length of subsequence having the same left and right rotations is - 4
Time complexity – O(N*10*10) ~ O(N) for traversing the string.
Space complexity – O(1) as we don’t use the extra space.
The first approach is time expensive as we find all subsequences of the given string. The second approach is one of the best approaches as it has the lowest time complexity. Programmers may try to find the longest substring having the same left and right rotation for more practice.