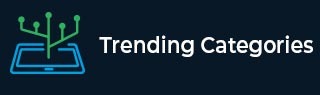
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python to Find number of lists in a tuple
A python tuple is ordered and unchangeable. But it can also be made up of lists as its elements. Given a tuple made up of lists, let’s find out how many lists are present in the tuple.
with len()
In this approach we will apply the len function. The len() function will give the count of lists which are the elements of the tuple.
Example
tupA = (['a', 'b', 'x'], [21,19]) tupB = (['n', 'm'], ['z','y', 'x'], [3,7,89]) print("The number of lists in tupA :\n" , len(tupA)) print("The number of lists in tupB :\n" , len(tupB))
Output
Running the above code gives us the following result −
The number of lists in tupA : 2 The number of lists in tupB : 3
Using a UDF
In case we have to use this operation again and again we can very well define a function which will check if the element we are passing is a tuple. Then apply len function to calculate the numbers of elements which is lists in it.
Example
tupA = (['a', 'b', 'x'], [21,19]) tupB = (['n', 'm'], ['z','y', 'x'], [3,7,89]) def getcount(tupl): if isinstance(tupl, tuple): return len(tupl) else: pass print("The number of lists in tupA :\n" , getcount(tupA)) print("The number of lists in tupA :\n" , getcount(tupB))
Output
Running the above code gives us the following result −
The number of lists in tupA : 2 The number of lists in tupA : 3
Advertisements