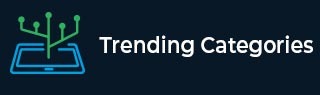
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program To Write Your Own atoi()
We are given a string that may represent a number and if it is a valid number then we have to convert it into an integer using the Python programming language. atoi() function is used in C programming language and used to convert the string which is passed as the parameter to it into an integer value if the string is a valid integer otherwise it shows the undefined behavior.
Sample Examples
Input 1
string S = "9834"
Output
9834
Explanation
We are given a string that represents a number so we have just got the same output.
Input 2
string S = "09 uy56"
Output
Invalid Input
Explanation
The given string is not a valid integer as it contains the whitespaces and lowercase English characters, so we have given the corresponding output.
Input 3
string str = "-987"
Output
-987
String is Valid
In this approach, we will assume the given string is the valid string and will not contain any whitespaces at the start, middle, or at end of the string.
This string contains only the digits and may contain a '-' character representing the number is the negative number.
In this approach, first, we will create a function that will take a single parameter and return the integer which is the answer.
For a number to be negative there will be a minus sign in front of it, which means we have to check if the character at the zeroth index is '-'' or not.
We will traverse over the string and will maintain a number to store the answer. At each index, we will multiply the current number by 10 to increase one decimal point and then add the current digit to it.
In the end, we will return the final answer, let us see the complete code:
Example
# function to converting the string to an integer def atoi(str): # Assuming the string is valid neg = 1 # checking for the negative number if (str[0] == '-'): neg = -1 ans = 0 i = 0 # if the number is the negative number then start from the next index if(neg == -1): i = i + 1 while (i < len(str)): cur = (int)(str[i]) ans = ans * 10 + cur i = i + 1 ans = ans* neg return ans; # returning the answer # defining the input and calling the function str = "-354663"; # calling the function ans = atoi(str) # printing the answer print("The value of the current number is:" , ans)
Output
The value of the current number is -354663
Time and Space Complexity
The time complexity of the above code is O(N), where N is the number of characters in the given string.
The space complexity of the above code is O(1), as we are not using any extra space.
String Can be Invalid
In this program, we will check if the current string can be invalid, so we will put a condition that if the string contains any character that is not in the range of the '0' to '9'. If any character occurs then we will return an Invalid string as the output otherwise we will follow the steps defined in the previous methods to get the output.
Also, we will use the ord function of the Python programming language to get the ASCII value of the current characters and add them to the number that stores the answer.
Example
# function for converting the string to an integer def atoi(str): # Assuming the string is valid neg = 1 # checking for the negative number if (str[0] == '-'): neg = -1 ans = 0 i = 0 # if the number is negative number then start from the next index if(neg == -1): i = i + 1 while (i < len(str)): # Checking for the base conditions # if the current character is not a digit return the invalid answer if((ord(str[i]) > ord('9')) or (ord(str[i]) < ord('0'))): print("The given string represents the invalid number") return cur = (int)(str[i]) ans = ans * 10 + cur i = i + 1 ans = ans* neg # printing the answer print("The value of the current number is:", ans); # defining the input and calling the function str = "-354 663"; # Calling the function atoi(str)
Output
The given string represents the invalid number
Time and Space Complexity
The time complexity of the above code is O(N), where N is the number of characters in the given string.
The space complexity of the above code is O(1), as we are not using any extra space.
Conclusion
In this tutorial, we have implemented a Python program to convert the number present in the form of a string to an integer. We have traversed over the string and checked if the current string represents a valid number or not. We have used the ord() python function to get the ASCII values of the characters and add them to the answer.