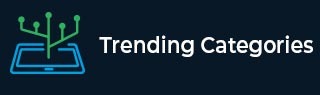
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python program to validate postal address format
Suppose we have a postal code we have to check whether it is valid or not. A valid postal code has following criteria
It must be a number in the range from 100000 to 999999 (both inclusive).
It must not contain more than one alternating repetitive digit pair.
So, if the input is like s = "700035", then the output will be True as this is in range 100000 to 999999 and there are no consecutive digits either.
To solve this, we will follow these steps −
- n := size of s
- nb := 0
- ok := True
- for i in range 0 to n - 1, do
- ok := ok and s[i] is a digit, then
- for i in range 0 to n-3, do
- nb := nb + (1 if s[i] is same as s[i+2] otherwise 0)
- return (true when ok is true and n is same as 6 and s[0] is not same as '0' and nb < 2), otherwise return false
Example
Let us see the following implementation to get better understanding
def solve(s): n = len(s) nb = 0 ok = True for i in range(n): ok = ok and s[i].isdigit() for i in range(n-2): nb += s[i] == s[i+2] return ok and n == 6 and s[0] != '0' and nb < 2 s = "700035" print(solve(s))
Input
"700035"
Output
True
Advertisements