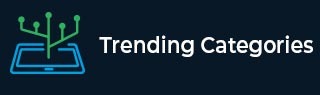
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program to trim a string from the left side
Python has various internal functions like isspace(), lstrip(), and replace() are used for trimming a given string. It means it will remove the whitespace from the left side of the string.
Let’s take an example to understand the trim of a string from the left side.
In the given string “MICROBOX” remove the left string MI and get the result value as “CROBOX”.
In the given string “synapse” remove the left string syn and get the result value as “apse”.
In this article, we will learn how to develop a Python program that will trim the string from the left side.
Syntax
The following syntax used in the examples are −
isspace()
This is a predefined method used in Python to allow the whitespace, newline, or space in a character.
lstrip("parameter as a string")
This is a predefined method used in Python and it accepts character as a parameter to remove the character of the string from the left side.
startswith()
This is an in-built method in Python that can be used to set the left side of a string for the identification of a given string.
Example 1
In this program, we will store the input string in the variable ‘str’. Then initialize the variable ‘i’ to the value 4 which will trim the total of 4 characters from the left side. Next, the variable ‘str’ iterate through the variable ‘char’ using for loop. Then use the if statement to search the whitespace by using isspace() method. If the space is not found in the string it will break the loop and the variable ‘i’ increment for each whitespace character. Now we are trimming the character by using str[i:] and storing the value in the variable ‘trim_str’. Finally, we are printing the result with the help of the variable ‘trim_str’.
#trim the string from left str = "My school" i = 4 for char in str: if not char.isspace(): break i += 1 trim_str = str[i:] #The use after slicing remove the left string. print("Trim the string of", i,"character from left:",trim_str)
Output
Trim the string of 4 character from left: chool
Example 2
In this program, we will store the input string in the variable ‘my_str’. Then create the new variable ‘trim_str’ to store the value by removing the trim character. The lstrip() method removes the character from the left side. Finally, we are printing the result with the help of the variable ‘trim_str’.
#Trim the string from left my_str = "SCHOOL" trim_str = my_str.lstrip("SC") print(trim_str)
Output
HOOL
Example 3
In this program, we will first store the input string in the variable str_name. Then set the string for left removal in the variable l_suffix. Now, start using an if-statement to check the condition of a given string with built-in function startswith() that will find the removal suffix. Next, use the slicing with len() method of the given string and store it in the variable str_name. Finally, print the result of the rest string in the variable str_name.
str_name = "asdfghjkl" l_suffix = "asd" if str_name.startswith(l_suffix): str_name = str_name[len(l_suffix):] print("After deleting the suffix from the left side:",str_name)
Output
After deleting the suffix from the left side: fghjkl
Example 4
In the following program, we will first store the input string in the variable str_name. Then set the left delete string in the variable del_suffix. Then use the if statement to check the condition for string removal with the help of built-in method startswith(). Next, use the method named replace() that accepts two parameters- l_suffix(delete) and an empty string “” to store the rest of the string. Finally, we are printing the result with the help of variable str_name.
str_name = "abcdefghi" l_suffix = "abcde" if str_name.startswith(l_suffix): str_name = str_name.replace(l_suffix, "") print("After deleting the suffix from the left side:",str_name)
Output
After deleting the suffix from the left side: fghi
Conclusion
We understood the difference between the two examples by trimming the string from the left side. We saw there are two different methods used in the examples which are isspace() and lstrip(). In example, 1 we used the after ‘:’ to remove the string from the left side.