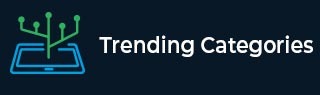
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python program to sort a list of tuples alphabetically
When it is required to sort a list of tuples in alphabetical order, the 'sort' method can be used. When using this, the contents of the original tuple get changed, since in-place sorting is performed.
The 'sort' function sorts the values in ascending order by default. If the order of sorting is specified as descending, it is sorted in descending order.
A list can be used to store heterogeneous values (i.e data of any data type like integer, floating point, strings, and so on).
A list of tuple basically contains tuples enclosed in a list.
Below is a demonstration of the same −
Example
def sort_tuple_vals(my_tup): my_tup.sort(key = lambda x: x[0]) return my_tup my_tup = [("Hey", 18), ("Jane", 33), ("Will", 56),("Nysa", 35), ("May", "Pink")] print("The tuple is ") print(my_tup) print("After sorting the tuple alphabetically, it becomes : ") print(sort_tuple_vals(my_tup))
Output
The tuple is [('Hey', 18), ('Jane', 33), ('Will', 56), ('Nysa', 35), ('May', 'Pink')] After sorting the tuple alphabetically, it becomes : [('Hey', 18), ('Jane', 33), ('May', 'Pink'), ('Nysa', 35), ('Will', 56)]
Explanation
- A function named 'sort_tuple_vals' is defined, that takes a tuple as a parameter.
- It uses the sort method and the lambda function to sort the elements in the tuple.
- This is returned when the function is called.
- Now the tuple is defined, and the function is called by passing this tuple as the parameter.
- The output is displayed on the console.
Advertisements