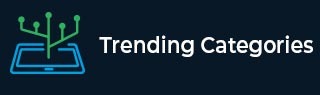
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python program to show diamond pattern with 2n-1 lines
Suppose we have a number n. We have to draw a diamond pattern with asterisks with 2n-1 lines. First 1 to n lines contain 1 to n number of asterisks, and next they are decreasing from n-1 to 1.
So, if the input is like n = 5, then the output will be
* * * * * * * * * * * * * * * * * * * * * * * * *
To solve this, we will follow these steps −
- for i in range 1 to n, do
- print a block '* ' i times and print it in justified format in center with (2*n-1) characters space in each line
- for i in range n-1 to 0, decrease by 1, do
- print a block '* ' i times and print it in justified format in center with (2*n-1) characters space in each line
Example
Let us see the following implementation to get better understanding
def solve(n): for i in range(1,n+1): print(('* '*i).center(2*n-1)) for i in range(n-1,0, -1): print(('* '*i).center(2*n-1)) n = 10 solve(n)
Input
10
Output
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
Advertisements