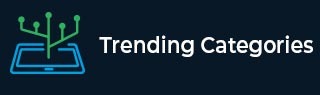
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program to remove the first given number of items from the array
An array is a data structure, which is used to store a set of elements of the same data type. And each element in the array is identified by an index value or key.
Arrays in Python
In Python doesn’t have a native array data structure. Instead, we can use List data structure to represent arrays.
[1, 2, 3, 4, 5]
Also we can use array or NumPy modules to work with arrays in python. An array defined by the array module is −
array('i', [1, 2, 3, 4])
A Numpy array defined by the NumPy module is −
array([1, 2, 3, 4])
Python indexing is starts from 0. And the above all arrays are indexed from starting 0 to (n-1).
Input Output Scenarios
Assume we have an integer array with 5 elements. And in output array the first few elements will be removed.
Input array: [1, 2, 3, 4, 5] Output: [3, 4, 5]
The first 2 elements 1, 2 are removed from the input array.
In this article, we will see how to remove the first given number of items from the array. Here we mainly use python slicing to remove the elements.
Slicing in python
Slicing allows accessing multiple elements at a time rather than accessing a single element with the indices.
Syntax
iterable_obj[start:stop:step]
Where,
Start: The starting index where slicing of the object starts. Default value is 0.
End: The ending index where slicing of the object stops. The Default value is len(object)-1.
Step: The number to increment the starting index. Default value is 1.
Using List
We can use list slicing to remove the first given number of elements from an array.
Example
Let’s take an example and apply list slicing to remove the first number of elements from an array.
# creating array lst = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] print ("The original array is: ", lst) print() numOfItems = 4 # remove first elements result = lst[numOfItems:] print ("The array after removing the elements is: ", result)
Output
The original array is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] The array after removing the elements is: [5, 6, 7, 8, 9, 10]
The first 4 elements are removed from the given array and the resultant array is stored in the result variable. In this example, the original array remains the same.
Example
By using the python del keyword and slicing object we can remove elements of an array.
# creating array lst = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] print ("The original array is: ", lst) print() numOfItems = 4 # remove first elements del lst[:numOfItems] print ("The array after removing the elements is: ", lst)
Output
The original array is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] The array after removing the elements is: [5, 6, 7, 8, 9, 10]
The statement lst[:numOfItems] retrieves the first given number of items of the array and the del keyword remove those items/elements.
Using NumPy array
Using the numpy module and slicing technique we can easily remove the number of items from an array.
Example
In this example, we will remove the first element from a numpy array.
import numpy # creating array numpy_array = numpy.array([1, 3, 5, 6, 2, 9, 8]) print ("The original array is: ", numpy_array) print() numOfItems = 3 # remove first elements result = numpy_array[numOfItems:] print ("The result is: ", result)
Output
The original array is: [1 3 5 6 2 9 8] The result is: [6 2 9 8]
We have successfully removed the first 2 elements from the numpy array using the array slicing.
Using array module
The array module in python also supports the indexing, and slicing techniques to access the elements.
Example
In this example, we will create an array using the array module.
import array # creating array arr = array.array('i', [2, 1, 4, 3, 6, 5, 8, 7]) print ("The original array is: ", arr) print() numOfItems = 2 # remove first elements result = arr[numOfItems:] print ("The result is: ", result)
Output
The original array is: array('i', [2, 1, 4, 3, 6, 5, 8, 7]) The result is: array('i', [4, 3, 6, 5, 8, 7])
The result array has removed first 2 elements from the array arr, here the array arr is unchanged.