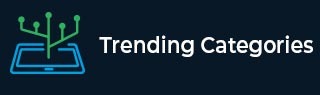
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program to Multiply two Matrices by Passing Matrix to a Function
A matrix is a two-dimensional array of many numbers arranged in rows and columns. And it is called as m X n matrix where m and n are the dimensions.
In general, the multiplication of two matrices can be possible only if the number of columns in the first matrix is equal to the number of rows in the second matrix.
Input Output Scenarios
Assuming we have two input matrices A and B having 3X3 rows and columns. Then the resultant matrix will also have 3 rows and 3 column.
[a, b, c] [j, k, l] [(a*j+b*m+c*p), (a*k+b*n+c*q), (a*l+b*o+c*r)] [d, e, f] * [m, n, o] = [(d*j+e*m+f*p), (d*k+e*n+f*q), (d*l+e*o+f*r)] [g, h, i] [p, q, r] [(g*j+h*m+i*p), (g*k+h*n+i*q), (g*l+h*o+i*r)]
The element-wise multiplication of matrices is explained below. In this case, the number of rows and columns of two input matrices must be the same.
[a, b, c] [j, k, l] [(a*j), (b*k), (c*i)] [d, e, f] * [m, n, o] = [(d*e), (e*n), (f*o)] [g, h, i] [p, q, r] [(g*p), (h*q), (i*r)]
Let’s Multiply two Matrices by Passing them to a Function
Using Loops
We will defined a user-define function using the def keyword in python to multiply two matrices. In the function, we declared a zero matrix that will store the resultant matrix. And by iterating the rows and columns of two matrices using the for loop we will multiply the elements of two matrices and store them in the result matrix.
Example
In the below example, we will multiply the two matrices by passing them to the user-defined function.
def multiply(A,B): result=[[0,0,0],[0,0,0],[0,0,0]] #for rows for i in range(len(A)): #for columns for j in range(len(B[0])): #for rows of matrix B for k in range(len(B)): result[i][j] += A[i][k] * B[k][j] for p in result: print(p) return #function for displaying matrix def display(matrix): for row in matrix: print(row) print() matrix_1 = [[2, 1, 2],[3, 2, 2], [1, 1, 2]] matrix_2 = [[1, 5, 3],[4, 2, 1], [1, 2, 2]] # Display two input matrices print('The first matrix is defined as:') display(matrix_1) print('The second matrix is defined as:') display(matrix_2) print("Result: ") multiply(matrix_1,matrix_2)
Output
The first matrix is defined as: [2, 1, 2] [3, 2, 2] [1, 1, 2] The second matrix is defined as: [1, 5, 3] [4, 2, 1] [1, 2, 2] Result: [8, 16, 11] [13, 23, 15] [7, 11, 8]
Here we will use some built-in Numpy functions to calculate the product of two matrices.
Using the Numpy.matmul() Function
The matmul() function will perform the matrix multiplication of two inputs passed to the function.
Example
Firstly we have Import the NumPy module and apply the np.matmul() function to calculate the product of the two matrices.
import numpy as np matrix_1 = np.array([[2, 1, 2],[3, 2, 2], [1, 1, 2]]) matrix_2 =np.array([[1, 5, 3],[4, 2, 1], [1, 2, 2]]) #function for displaying matrix def display(matrix): for row in matrix: print(row) print() # Display two input matrices print('The first matrix is defined as:') display(matrix_1) print('The second matrix is defined as:') display(matrix_2) print("Result: ") print(np.matmul(matrix_1 ,matrix_2))
Output
The first matrix is defined as: [2 1 2] [3 2 2] [1 1 2] The second matrix is defined as: [1 5 3] [4 2 1] [1 2 2] Result: [[ 8 16 11] [13 23 15] [ 7 11 8]]
Using the Numpy.multiply() Function
It will perform the element-wise multiplication operation of two input matrices.
Example
Initially, We will create the two matrices matrix using the numpy.array() function. And by using the np.multiply() function we will calculate the element-wise multiplication of two matrices.
import numpy as np matrix_1 = np.array([[2, 1, 2],[3, 2, 2], [1, 1, 2]]) matrix_2 =np.array([[1, 5, 3],[4, 2, 1], [1, 2, 2]]) #function for displaying matrix def display(matrix): for row in matrix: print(row) print() # Display two input matrices print('The first matrix is defined as:') display(matrix_1) print('The second matrix is defined as:') display(matrix_2) print("Result: ") print(np.multiply(matrix_1 ,matrix_2))
Output
The first matrix is defined as: [2 1 2] [3 2 2] [1 1 2] The second matrix is defined as: [1 5 3] [4 2 1] [1 2 2] Result: [[ 2 5 6] [12 4 2] [ 1 2 4]]
Using the Numpy.dot() Function
The dot() function will perform the dot product on two inputs matrices.
Example
We will give two numpy arrays to the np.dot() function, to perform the dot product of both the inputs.
import numpy as np matrix_1 = np.array([[2, 1, 2],[3, 2, 2], [1, 1, 2]]) matrix_2 =np.array([[1, 5, 3],[4, 2, 1], [1, 2, 2]]) #function for displaying matrix def display(matrix): for row in matrix: print(row) print() # Display two input matrices print('The first matrix is defined as:') display(matrix_1) print('The second matrix is defined as:') display(matrix_2) print("Result: ") print(np.dot(matrix_1 ,matrix_2))
Output
The first matrix is defined as: [2 1 2] [3 2 2] [1 1 2] The second matrix is defined as: [1 5 3] [4 2 1] [1 2 2] Result: [[ 8 16 11] [13 23 15] [ 7 11 8]]