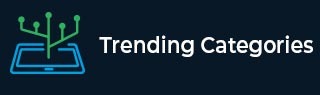
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python program to get maximum of each key Dictionary List
When it is required to get the maximum of each key in a list of dictionary elements, a simple iteration is used.
Example
Below is a demonstration of the same
my_list = [{"Hi": 18, "there": 13, "Will": 89}, {"Hi": 53, "there": 190, "Will": 87}] print("The list is : ") print(my_list) my_result = {} for elem in my_list: for key, val in elem.items(): if key in my_result: my_result[key] = max(my_result[key], val) else: my_result[key] = val print("The result is : ") print(my_result)
Output
The list is : [{'Will': 89, 'there': 13, 'Hi': 18}, {'Will': 87, 'there': 190, 'Hi': 53}] The result is : {'Will': 89, 'there': 190, 'Hi': 53}
Explanation
A list of dictionary is defined and is displayed on the console.
An empty dictionary is defined.
The list is iterated over, and the elements are accessed.
If the key is present in the previously defined dictionary, the maximum of the key and the value is determined, and stored in the ‘key’ index of the dictionary.
Otherwise, the value is stored in the ‘key’ index of the dictionary.
This is displayed as the output on the console.
Advertisements