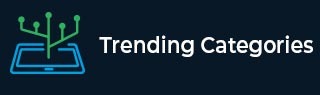
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program to Find Element Occurring Odd Number of Times in a List
When it is required to find an element that occurs odd number of times in a list, a method can be defined. This method iterates through the list and checks to see if the elements in the nested loops match. If they do, the counter is incremented. If that count is not divisible by 2, the specific element of the list is returned as the result. Otherwise, -1 is returned as the result.
Below is a demonstration of the same −
Example
def odd_occurence(my_list, list_size): for i in range(0, list_size): count = 0 for j in range(0, list_size): if my_list[i] == my_list[j]: count+= 1 if (count % 2 != 0): return my_list[i] return -1 my_list = [34, 56, 78, 99, 23, 34, 34, 56, 78, 99, 99, 99, 99, 34, 34, 56, 56 ] print("The list is :") print(my_list) n = len(my_list) print("The length is :") print(n) print("The method to find the element that occurs odd number of times is called ") print("The element that occurs odd number of times is :") print(odd_occurence(my_list, n))
Output
The list is : [34, 56, 78, 99, 23, 34, 34, 56, 78, 99, 99, 99, 99, 34, 34, 56, 56] The length is : 17 The method to find the element that occurs odd number of times is called The element that occurs odd number of times is : 34
Explanation
A method named ‘odd_occurence’ is defined, which takes the list and its size as parameters.
The listed size is taken as the range and the list is iterated over.
Two nested loops are iterated, and if the element in the list matches the first and second loop iterations, the ‘count’ variable is incremented.
If the ‘count’ variable is an odd number, the specific element in the list is returned.
A list of integers is defined and is displayed on the console.
The length of the list is stored in a variable.
The method is called by passing relevant parameters.
The output is displayed on the console.