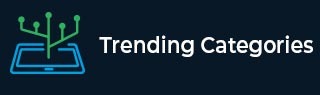
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program to Extract Strings with a digit
In this article, we will learn how to extract strings with a digit in python.
Methods Used
The following are the various methods to accomplish this task −
Using list comprehension, any() and isdigit() functions
Using any(), filter() and lambda functions
Without using any built-in functions
Using ord() function
Example
Assume we have taken an input list containing strings. We will now extract strings containing a digit in an input list using the above methods.
Input
inputList = ['hello562', 'tutorialspoint', 'python20', 'codes', '100']
Output
List of Strings containing any digits: ['hello562', 'python20', '100']
In the above input list, the elements 'hello562', 'python20', '100' contain digits. Hence they are extracted from the input list.
Method 1: Using list comprehension, any() and isdigit() functions
Algorithm (Steps)
Following are the Algorithm/steps to be followed to perform the desired task –.
Create a variable to store the input list of strings
Print the input list.
Use the List comprehension to traverse through input list elements and check whether the string contains any digit character by character using any() function(returns True if any item in an iterable is true, else returns False) and isdigit() function
isdigit() function − If all of the characters are digits, the isdigit() method returns True; otherwise, it returns False.
Print the resultant list of Strings containing any digits.
Example
The following program returns a list that extracts the strings containing digits in an input list using list comprehension, any(), and isdigit() functions –
# input list of strings inputList = ['hello562', 'tutorialspoint', 'python20', 'codes', '100'] # printing input list print("Input list:", inputList) # traversing through list elements and checking if the string # contains any digit character outputList = [item for item in inputList if any(c for c in item if c.isdigit())] # printing resultant list of Strings containing any digits print("List of Strings containing any digits:\n", outputList)
Output
On executing, the above program will generate the following output –
Input list: ['hello562', 'tutorialspoint', 'python20', 'codes', '100'] List of Strings containing any digits: ['hello562', 'python20', '100']
Method 2: Using any(), filter() and lambda functions
filter() function − filters the specified sequence using a function that determines if each element in the sequence is to be true or false.
Lambda Function
A lambda function is an anonymous function that is small.
A lambda function can have an unlimited/any number of arguments but only one expression.
Syntax
lambda arguments : expression
Example
The following program returns a list that extracts the strings containing digits in an input list using any(), filter(), and lambda functions –
# input list of strings inputList = ['hello562', 'tutorialspoint', 'python20', 'codes', '100'] # printing input list print("Input list:", inputList) # filtering the list elements if any string character is a digit outputList = list(filter(lambda s: any( e for e in s if e.isdigit()), inputList)) # printing resultant list of Strings containing any digits print("List of Strings containing any digits:\n", outputList)
Output
On executing, the above program will generate the following output –
Input list: ['hello562', 'tutorialspoint', 'python20', 'codes', '100'] List of Strings containing any digits: ['hello562', 'python20', '100']
Method 3: Without using any built-in functions
replace() function − returns a copy of the string that replaces all occurrences of an old substring with another new substring.
Syntax
string.replace(old, new, count)
Example
The following program returns a list that extracts the strings containing digits in an input list without using any built-in functions –
# function that returns string with digits by accepting input string as an argument def newFunction(str): # initializing all digits digitsStr= "0123456789" # storing the string passed to the function in a temp variable temp = str # traversing through each digit in the above digits string for d in digitsStr: # replacing that digit with space str = str.replace(d, "") # checking whether the length of the temp variable string is not equal to the passed string if(len(temp) != len(str)): # returning true if the condition is true return True # else returning false return False # input list of strings inputList = ['hello562', 'tutorialspoint', 'python20', 'codes', '100'] # printing input list print("Input list:", inputList) # empty list for storing list elements containing digits outputList = [] # traversing through each element of the input list for element in inputList: # checking whether the above defined newFunction() returns true if newFunction(element): # appending that element to the output list outputList.append(element) # printing resultant list of Strings containing any digits print("List of Strings containing any digits:\n", outputList)
Output
On executing, the above program will generate the following output –
Input list: ['hello562', 'tutorialspoint', 'python20', 'codes', '100'] List of Strings containing any digits: ['hello562', 'python20', '100']
Method 4: Using the ord() function
ord() function − returns the Unicode code of a given character as a number.
Example
The following program returns a list that extracts the strings containing digits in an input list without using the ord() function –
# input list of strings inputList = ['hello562', 'tutorialspoint', 'python20', 'codes', '100'] # printing input list print("Input list:", inputList) # empty list for storing list elements containing digits outputList = [] # traversing through each element of the input list for element in inputList: # traversing through each character of the current element for c in element: # checking whether the ASCII value of char is greater than or # equal to 0 and less than or equal to 9 if(ord(c) >= ord('0') and ord(c) <= ord('9')): # appending that element to output list outputList.append(element) # breaking the loop break # printing resultant list of Strings containing any digits print("List of Strings containing any digits:\n", outputList)
Output
On executing, the above program will generate the following output –
Input list: ['hello562', 'tutorialspoint', 'python20', 'codes', '100'] List of Strings containing any digits: ['hello562', 'python20', '100']
Conclusion
From the given list of strings, we have learned 4 different ways to extract string elements containing digits. We also learned how to filter the list according to the condition. We also learned that, rather than using nested lists, any() method inside the lambda function may be used to apply conditions.