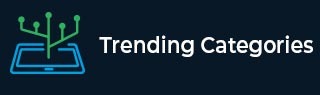
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program to Display Fibonacci Sequence Using Recursion
When it is required to print the fibonacci sequence using the method of recursion, a method can be declared that calls the same method again and again until a base value is reached.
Below is a demonstration of the same −
Example
def fibonacci_recursion(my_val): if my_val <= 1: return my_val else: return(fibonacci_recursion(my_val-1) + fibonacci_recursion(my_val-2)) num_terms = 12 if num_terms <= 0: print("Enter a positive integer") else: print("The fibonacci sequence is :") for i in range(num_terms): print(fibonacci_recursion(i))
Output
The fibonacci sequence is : 0 1 1 2 3 5 8 13 21 34 55 89
Explanation
A method named ‘fibonacci_recursion’ is defined that takes a value as parameter.
If the value is less than one, it is returned as output.
Otherwise the same method is called again and again until a base condition is reached.
The number of terms in the Fibonacci sequence is defined.
The method is called, and the output is displayed on the console.
Advertisements