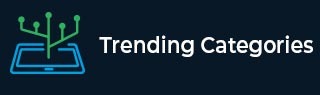
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program To Determine If a Given Matrix is a Sparse Matrix
A matrix is a rectangular array where the set of numbers arranged in rows and columns. And it is called as an m X n matrix where m and n are the dimensions.
If a matrix contains a very less number of non-zero elements compared to the zero elements then it is called a sparse matrix.
[0, 0, 3, 0, 0] [0, 1, 0, 0, 6] [1, 0, 0, 9, 0] [0, 0, 2, 0, 0]
The above matrix is 4X5 matrix here most of the numbers are zero. Only a few elements are non-zero so that we can it a sparse matrix.
To check whether the given matrix is a sparse matrix or not, we need to compare the total number of elements and zeros. If the count of zero elements is more than half of the elements in the matrix. Then we can call the given matrix a sparse matrix.
(m * n)/2
Let’s discuss the different approaches to find if the given matrix is a sparse matrix or not.
Using For Loop
Using the for loop we can easily iterate the array elements in python.
Example
Initially, we will iterate the matrix rows and count the number of zeroes present in each row. Then the count value will be stored in the counter variable.
After that we will compare the value in counter variable with half of the elements in the matrix to determine if the given matrix is a sparse matrix or not.
def isSparse(array, m, n): counter = 0 # Count number of zeros for i in range(0, m): for j in range(0, n): if (array[i][j] == 0): counter = counter + 1 return (counter > ((m * n) // 2)) arr = [[0, 0, 3], [0, 0, 0], [1, 8, 0]] print("The original matrix: ") for row in arr: print(row) print() # check if the given matrix is sparse matrix or not if (isSparse(arr, len(arr), len(arr[0]))): print("The given matrix is a sparse matrix") else: print("The given matrix is not a sparse matrix")
Output
The original matrix: [0, 0, 3] [0, 0, 0] [1, 8, 0] The given matrix is a sparse matrix
The above matrix is a sparse matrix.
Example
In this example, we will use the list.count() method to count the zero elements of each row in the loop and store the count in the counter variable.
def isSparse(array, m, n): counter = 0 # Count number of zeros for i in array: counter += i.count(0) return (counter > ((m * n) // 2)) arr = [[0, 0, 3], [0, 0, 0], [1, 8, 0]] print("The original matrix: ") for row in arr: print(row) print() # check if the given matrix is sparse matrix or not if (isSparse(arr, len(arr), len(arr[0]))): print("The given matrix is a sparse matrix") else: print("The given matrix is not a sparse matrix")
Output
The original matrix: [0, 0, 3] [0, 0, 0] [1, 8, 0] The given matrix is a sparse matrix
Using the SciPy Library
By using the SciPy library in Python we can create sparse matrices. In the below example, we have used the csr_matrix() function to create a sparse matrix in compressed sparse row format.
And the issparse() function is used to check whether the given object is a sparse matrix or not.
Example
Initially, we will create an array using the nested list then convert it to the sparse matrix using the csr_matrix() method.
from scipy.sparse import issparse, csr_matrix arr = [[0, 0, 3], [0, 0, 0], [1, 8, 0]] matrix = csr_matrix(arr) print("The original matrix: ") print(matrix) print() # check if the given matrix is sparse matrix or not if (issparse(matrix)): print("The given matrix is a sparse matrix") else: print("The given matrix is not a sparse matrix")
Output
The original matrix: (0, 2) 3 (2, 0) 1 (2, 1) 8 The given matrix is a sparse matrix
The csr_matrix() method only stores the data points (non zero elements) in memory.
Note − The issparse() method has nothing to do with how many elements the input matrix has. Rather, it checks if a given object is an instance of a spmatrix or not.