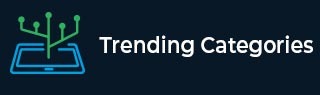
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python program to convert a list to a set based on a common element
When it is required to convert a list to a set based on a specific common element, a method can be defined that iterates through the set using ‘enumerate’ and places a specific condition on the elements. The ‘union’ method and the ‘map’ methods are used.
Example
Below is a demonstration of the same
def common_elem_set(my_set): for index, val in enumerate(my_set): for j, k in enumerate(my_set[index + 1:], index + 1): if val & k: my_set[index] = val.union(my_set.pop(j)) return common_elem_set(my_set) return my_set my_list = [[18, 14, 12, 19], [9, 6, 2, 1], [54, 32, 21, 17], [18, 11, 13, 12]] print("The list is :") print(my_list) my_set = list(map(set, my_list)) my_result = common_elem_set(my_set) print("The result is :") print(my_result)
Output
The list is : [[18, 14, 12, 19], [9, 6, 2, 1], [54, 32, 21, 17], [18, 11, 13, 12]] The result is : [{11, 12, 13, 14, 18, 19}, {9, 2, 6, 1}, {32, 17, 21, 54}]
Explanation
A method named ‘common_elem_set’ is defined which takes a list as parameter.
The list is iterated over using enumerate.
A condition is set using ‘&’ and if it is fulfilled, the output is returned.
Outside the method, a list of list is defined.
It is displayed on the console.
It is converted to a set using the ‘map’ method, and then converted to a list again.
This is assigned to a variable.
Now the method is called by passing this variable.
The output is displayed on the console.