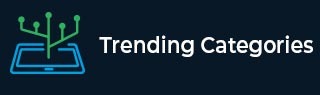
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program to convert a list into matrix with size of each row increasing by a number
When it is required to convert a list into matrix with the size of every row increasing by a number, the ‘//’ operator and a simple iteration is used.
Example
Below is a demonstration of the same
my_list = [42, 45, 67, 89, 99, 10, 23, 12, 31, 43, 60, 1, 0] print("The list is :") print(my_list) my_key = 3 print("The value of key is ") print(my_key) my_result = [] for index in range(0, len(my_list) // my_key): my_result.append(my_list[0: (index + 1) * my_key]) print("The resultant matrix is :") print(my_result)
Output
The list is : [42, 45, 67, 89, 99, 10, 23, 12, 31, 43, 60, 1, 0] The value of key is 3 The resultant matrix is : [[42, 45, 67], [42, 45, 67, 89, 99, 10], [42, 45, 67, 89, 99, 10, 23, 12, 31], [42, 45, 67, 89, 99, 10, 23, 12, 31, 43, 60, 1]]
Explanation
A list is defined and is displayed on the console.
A value for key is defined and is displayed on the console.
An empty list is created.
A simple iteration is used along with ‘//’ operator, and the element from a specific index multiplied with key.
This is appended to the empty list.
This list is displayed as output on the console.
Advertisements