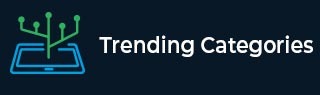
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Program to Compute the Value of Euler's Number e. Use the Formula: e = 1 + 1/1! + 1/2! + …… 1/n!
When it is required to implement Euler’s number, a method is defined, that computes the factorial.
Another method is defined that find the sum of these factorial numbers.
Below is the demonstration of the same −
Example
def factorial_result(n): result = 1 for i in range(2, n + 1): result *= i return result def sum_result(n): s = 0.0 for i in range(1, n + 1): s += 1.0 / factorial_result(i) print(s) my_value = 5 print("The value is :") print(my_value) print("The result is :") sum_result(my_value)
Output
The value is : 5 The result is : 1.7166666666666668
Explanation
A method named ‘factorial_result’ is defined, that takes an integer ‘n’ as parameter that computes the factorial of a given number.
Another method named ‘sum_result’ is defined, that takes integer ‘n’ as parameter that iterates over a range of numbers and adds the numbers.
Outside the methods, a value is defined, and the ‘sum_value’ is called by passing this value as parameter.
The output computed is displayed on the console.
Advertisements