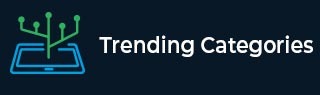
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Pandas - Return numpy array of python datetime.time objects with timezone information
To return numpy array of python datetime.time objects with timezone information, use the datetimeindex.timetz property.
At first, import the required libraries −
import pandas as pd
Create a DatetimeIndex with period 5 and frequency as us i.e. nanoseconds −
datetimeindex = pd.date_range('2021-10-20 02:30:50', periods=5, tz='Australia/Sydney', freq='ns')
Display DateTimeIndex −
print("DateTimeIndex...\n", datetimeindex)
Returns only the time part of Timestamp with timezone information −
print("\nThe numpy array (time part with timezone)..\n",datetimeindex.timetz)
Example
Following is the code −
import pandas as pd # DatetimeIndex with period 5 and frequency as us i.e. nanoseconds # The timezone is Australia/Sydney datetimeindex = pd.date_range('2021-10-20 02:30:50', periods=5, tz='Australia/Sydney', freq='ns') # display DateTimeIndex print("DateTimeIndex...\n", datetimeindex) # Returns only the time part of Timestamp with timezone information print("\nThe numpy array (time part with timezone)..\n",datetimeindex.timetz)
Output
This will produce the following code −
DateTimeIndex... DatetimeIndex([ '2021-10-20 02:30:50+11:00', '2021-10-20 02:30:50.000000001+11:00', '2021-10-20 02:30:50.000000002+11:00', '2021-10-20 02:30:50.000000003+11:00', '2021-10-20 02:30:50.000000004+11:00'], dtype='datetime64[ns, Australia/Sydney]', freq='N') The numpy array (time part with timezone).. [datetime.time(2, 30, 50, tzinfo=<DstTzInfo 'Australia/Sydney' AEDT+11:00:00 DST>) datetime.time(2, 30, 50, tzinfo=<DstTzInfo 'Australia/Sydney' AEDT+11:00:00 DST>) datetime.time(2, 30, 50, tzinfo=<DstTzInfo 'Australia/Sydney' AEDT+11:00:00 DST>) datetime.time(2, 30, 50, tzinfo=<DstTzInfo 'Australia/Sydney' AEDT+11:00:00 DST>) datetime.time(2, 30, 50, tzinfo=<DstTzInfo 'Australia/Sydney' AEDT+11:00:00 DST>)]
Advertisements