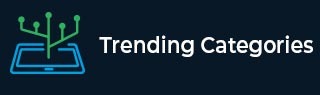
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python β numpy.reshape
numpy.reshape() gives a new shape to an array without changing its data. Its syntax is as follows −
numpy.reshape(arr, newshape, order='C')
Parameters
numpy.reshape() can accept the following parameters −
arr − Input array.
shape − endpoint of the sequence
newshape − If an integer, then the result it will be a 1-D array of that length, and one dimension can be -1.
order − It defines the order in which the input array elements should be read.
If the order is ‘C’, then it reads and writes the elements which are using a C-like index order where the last index changes the fastest and the first axis index changes slowly.
‘F’ means to read and write the elements using a Fortran-like index order where the last index axis changes slowly and the first axis index changes fast.
‘A’ means to read/write the elements in Fortran-like index order, when the array is contiguous in memory.
Example 1
Let us consider the following example −
# Import numpy import numpy as np # input array x = np.array([[3,5,6], [7,8,9]]) print("Array Input :\n", x) # reshape() function y = np.reshape(x, (3, -3)) print("Reshaped Array: \n", y)
Output
It will generate the following output −
Array Input : [[3 5 6] [7 8 9]] Reshaped Array: [[3 5] [6 7] [8 9]]
Example 2
Let us take another example −
# Import numpy import numpy as np # Create an input array x = np.array([[1,3,4], [4,6,7]]) print("Array Input :\n", x) # reshape() function y = np.reshape(x, 6, order='C') print("Reshaped Array: \n", y)
Output
It will generate the following output −
Array Input : [[1 3 4] [4 6 7]] Reshaped Array: [1 3 4 4 6 7]