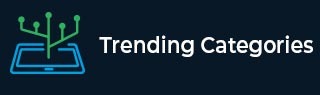
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python – List Elements Grouping in Matrix
When it is required to list elements grouping in a matrix, a simple iteration, the ‘pop’ method, list comprehension and ‘append’ methods are used.
Example
Below is a demonstration of the same −
my_list = [[14, 62], [51, 23], [12, 62], [78, 87], [41, 14]] print("The list is :") print(my_list) check_list = [14, 12, 41, 62] print("The list is :") print(check_list) my_result = [] while my_list: sub_list_1 = my_list.pop() sub_list_2 = [element for element in check_list if element not in sub_list_1] try: my_list.remove(sub_list_2) my_result.append([sub_list_1, sub_list_2]) except ValueError: my_result.append(sub_list_1) print("The result is :") print(my_result)
Output
The list is : [[14, 62], [51, 23], [12, 62], [78, 87], [41, 14]] The list is : [14, 12, 41, 62] The result is : [[[41, 14], [12, 62]], [78, 87], [51, 23], [14, 62]]
Explanation
A list of list of integers is defined and is displayed on the console.
Another list of integers is defined and displayed on the console.
An empty list is defined.
A simple iteration is used, and the top most element is popped using ‘pop’ method.
This is assigned to a variable ‘sub_list_1’.
A list comprehension is used to iterate over the second list, and check if element is not there in ‘sub_list_1’.
The ‘try’ and ‘except’ block is used to append specific elements to the empty list.
This list is displayed as the output on the console.
Advertisements