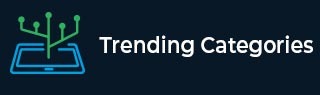
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python - Filter even values from a list
As part of data analysis require to filter out values from a list meeting certain criteria. In this article we'll see how to filter out only the even values from a list.
We have to go through each element of the list and divide it with 2 to check for the remainder. If the remainder is zero then we consider it as an even number. After fetching these even numbers from a list we will put a condition to create a new list which excludes this even numbers. That new list is the result of the filtering condition we applied.
Using for Loop
This is the simplest way to read element of the list and check for divisibility with 2. The below code contains for loop which reads the elements and checks for divisibility by 2. Then it appends the correct element to an empty list, which will be the result of the filtering condition.
Example
list_A = [33, 35, 36, 39, 40, 42] res = [] for n in list_A: if n % 2 == 0: res.append(n) print(res)
Output
Running the above code gives us the following result:
[36, 40, 42]
Using While Loop
When we have lists of unknown lengths, we can use a while loop and the len() function to achieve the same thing which was there in the above program.
Example
list_A = [33, 35, 36, 39, 40, 42] res = [] n = 0 while (n < len(list_A)): if list_A[n] % 2 == 0: res.append(list_A[n]) n += 1 print(res)
Output
Running the above code gives us the following result:
[36, 40, 42]
Using filter()
We can also use a combination of lambda and filter function. The items picked by lambda function can be filtered out from the original list by applying the filter function.
Example
list_A = [33, 35, 36, 39, 40, 42] res = [] x = lambda m: m % 2 == 0 res = list(filter(x, list_A)) print(res)
Output
Running the above code gives us the following result:
[36, 40, 42]