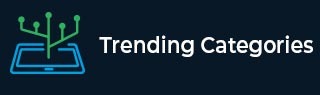
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python - Compute last of group values in a Pandas DataFrame
To compute last of group values, use the groupby.last() method. At first, import the required library with an alias −
import pandas as pd;
Create a DataFrame with 3 columns −
dataFrame = pd.DataFrame( { "Car": ['BMW', 'Lexus', 'BMW', 'Tesla', 'Lexus', 'Tesla'],"Place": ['Delhi','Bangalore','Pune','Punjab','Chandigarh','Mumbai'],"Units": [100, 150, 50, 80, 110, 90] } )
Now, group DataFrame by a column −
groupDF = dataFrame.groupby("Car")
Compute last of group values and resetting index −
res = groupDF.last() res = res.reset_index()
Example
Following is the complete code. The last occurrence of repeated values are displayed i.e. last of group values −
import pandas as pd; dataFrame = pd.DataFrame( { "Car": ['BMW', 'Lexus', 'BMW', 'Tesla', 'Lexus', 'Tesla'],"Place": ['Delhi','Bangalore','Pune','Punjab','Chandigarh','Mumbai'],"Units": [100, 150, 50, 80, 110, 90] } ) print"DataFrame ...\n",dataFrame # grouping DataFrame by column Car groupDF = dataFrame.groupby("Car") res = groupDF.last() res = res.reset_index() print"\nLast of group values = \n",res
Output
This will produce the following output −
DataFrame ... Car Place Units 0 BMW Delhi 100 1 Lexus Bangalore 150 2 BMW Pune 50 3 Tesla Punjab 80 4 Lexus Chandigarh 110 5 Tesla Mumbai 90 Last of group values = Car Place Units 0 BMW Pune 50 1 Lexus Chandigarh 110 2 Tesla Mumbai 90
Advertisements