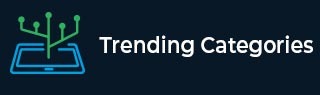
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python – Check alternate peak elements in List
When it is required to check the alternate peak elements in a list, a function is defined that iterates through the list, the adjacent elements of the array are compared and depending on this, the output is displayed on the console.
Example
Below is a demonstration of the same
def find_peak(my_array, array_length) : if (array_length == 1) : return 0 if (my_array[0] >= my_array[1]) : return 0 if (my_array[array_length - 1] >= my_array[array_length - 2]) : return array_length - 1 for i in range(1, array_length - 1) : if (my_array[i] >= my_array[i - 1] and my_array[i] >= my_array[i + 1]) : return i my_list = [ 1, 3, 20, 4, 1, 0 ] list_length = len(my_list) print("The list is :") print(my_list) print("The result is") print(find_peak(my_array, array_length))
Output
The list is : [1, 3, 20, 4, 1, 0] The result is 2
Explanation
A method named ‘find_peak’ is defined that takes the list and its length as parameters.
It checks the length of the list and returns result depending on that.
The adjacent elements of the list are compared and final result is returned.
Outside the method, a list is defined, and is displayed on the console.
The length of the list is assigned to a variable.
The method is called by passing the required parameter.
The result is displayed on the console.
Advertisements