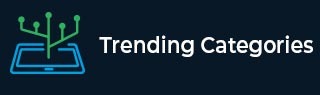
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python – Assign Alphabet to each element
When it is required to assign an alphabet to every element of an integer list, the ‘ascii_lowercase’ method, and the list comprehension are used.
Example
Below is a demonstration of the same −
import string my_list = [11, 51, 32, 45, 21, 66, 12, 58, 90, 0] print("The list is : " ) print(my_list) print("The list after sorting is : " ) my_list.sort() print(my_list) temp_val = {} my_counter = 0 for element in my_list: if element in temp_val: continue temp_val[element] = string.ascii_lowercase[my_counter] my_counter += 1 my_result = [temp_val.get(element) for element in my_list] print("The resultant list is : ") print(my_result)
Output
The list is : [11, 51, 32, 45, 21, 66, 12, 58, 90, 0] The list after sorting is : [0, 11, 12, 21, 32, 45, 51, 58, 66, 90] The resultant list is : ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j']
Explanation
The required packages are imported into the environment.
A list of integers is defined and is displayed on the console.
It is sorted using the ‘sort’ method and is displayed on the console again.
An empty dictionary is defined.
A counter is initialized to 0.
It is iterated over, and the ‘continue’ operator is used when a condition is satisfied.
Otherwise, the ‘ascii_lowercase’ method is used and assigned to a specific index in the dictionary.
A list comprehension is used to iterate over the list and uses ‘get’ method.
The elements from this are stored in a list and assigned to a variable.