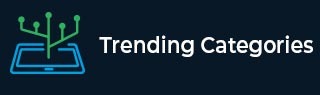
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to reverse the position of each word of a given string in Python
Suppose we have a string of words delimited by spaces; we have to reverse the order of words.
So, if the input is like "Hello world, I love python programming", then the output will be "programming python love I world, Hello"
To solve this, we will follow these steps −
- temp := make a list of words by splitting s using blank space
- temp := reverse the list temp
- return a string by joining the elements from temp using space delimiter.
Let us see the following implementation to get better understanding −
Example
class Solution: def solve(self, s): temp = s.split(' ') temp = list(reversed(temp)) return ' '.join(temp) ob = Solution() sentence = "Hello world, I love python programming" print(ob.solve(sentence))
Input
"Hello world, I love python programming"
Output
programming python love I world, Hello
Advertisements