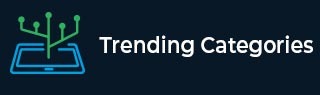
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to reset a polygon to its initial state in Python
Suppose, there is a polygon with n vertices, n flipping axis, and n rotation points. The following are true for the flipping axes and rotation points
- If n is odd, each flipping axis passes through only one vertex and the middle of the opposite side.
- If n is even, half of the axes pass through a pair of opposite vertex and the other half passes through a pair of opposite sides.
- Two following axes have an angle of 360/2n.
Now, we rotate the polygon provided. We have n different types of rotators, a k-rotator rotates the polygon at axis k clockwise by (360 x k)/n degrees. There is a list input list that contains several pairs of integers. The first integer of a pair represents if the polygon is to beflipped or rotated. If the first integer is 1 then the polygon is rotated, if it is 2 the polygon is flipped. The second integer is k, if the polygon is flipped it is flipped at axis k or otherwise, if it is rotated it is rotated by an angle of 360/2n. The rotations and flipping are then done while the list is not empty.
Our task here is to add another element to the list so that the polygon can be reset to its initial position.
The image specifies the rotation axes of two types of polygons.
So, if the input is like n = 6, input_list = [[1, 2], [1, 4], [2, 3], [2, 5], [1, 6]], then the output will be (1, 4)
After the transformations, a rotation along the 4th axis will reset the polygon to its initial position.
To solve this, we will follow these steps −
- decision_var := False
- position := 0
- for each item in input_list, do
- x := item[0]
- y := item[1]
- if x is same as 1, then
- position := position + y
- otherwise
- position := y - position
- decision_var := not(decision_var)
- position := position mod n
- if decision_var is non-zero, then
- return a pair (2, position)
- otherwise,
- return a pair (1, n - position)
Example
Let us see the following implementation to get better understanding −
def solve(n, input_list): decision_var = False position = 0 for item in input_list: x = item[0] y = item[1] if x == 1: position += y else: position = y - position decision_var = not decision_var position = position % n if decision_var: return (2, position) else: return (1, n - position) print(solve(6, [[1, 2], [1, 4], [2, 3], [2, 5], [1, 6]]))
Input
6, [[1, 2], [1, 4], [2, 3], [2, 5], [1, 6]]
Output
(1, 4)