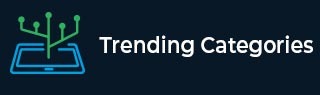
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to maximize number of nice divisors in Python
Suppose we have a number pf represents number of prime factors. We have to make a positive number n that satisfies the following conditions −
The number of prime factors of n (may or may not be distinct) is at most pf.
The number of nice divisors of n is maximized. As we know a divisor of n is nice when it is divisible by every prime factor of n.
We have to find the number of nice divisors of n. If the answer is too large then return result modulo 10^9 + 7.
So, if the input is like pf = 5, then the output will be 6 because for n = 200 we have prime factors [2,2,2,5,5] and its nice divisors are [10,20,40,50,100,200] so 6 divisors.
To solve this, we will follow these steps −
if pf is same as 1, then
return 1
m := 10^9 + 7
q := quotient of pf/3, r := pf mod 3
if r is same as 0, then
return 3^q mod m
otherwise when r is same as 1, then
return (3^(q-1) mod m)*4 mod m
otherwise,
return (3^q mod m)*2 mod m
Example
Let us see the following implementation to get better understanding
def solve(pf): if pf == 1: return 1 m = 10** 9 + 7 q, r = divmod(pf, 3) if r == 0: return pow(3, q, m) elif r == 1: return pow(3, q-1, m) * 4 % m else: return pow(3, q, m) * 2 % m pf = 5 print(solve(pf))
Input
5
Output
6