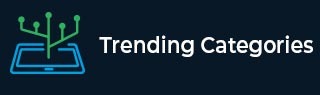
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to make the XOR of all segments equal to zero in Python
Suppose we have an array called nums and another value k. The XOR of a segment [left, right] (left <= right) is the XOR of all elements whose indices are in between left and right (inclusive).
We have to find the minimum number of elements to change in the array such that the XOR of all segments of size k is same as zero.
So, if the input is like nums = [3,4,5,2,1,7,3,4,7], k = 3, then the output will be 3 because we can modify elements at indices 2, 3, 4 to make the array [3,4,7,3,4,7,3,4,7].
To solve this, we will follow these steps −
LIMIT := 1024
temp := make an array whose size is LIMIT x k, and fill with 0
for each index i and value x in nums, do
temp[i mod k, x] := temp[i mod k, x] + 1
dp := an array of size LIMIT and fill with -2000
dp[0] := 0
for each row in temp, do
maxprev := maximum of dp
new_dp := an array of size LIMIT and fill with maxprev
for each index i and value cnt row, do
if cnt > 0, then
for each index j and value prev in dp, do
new_dp[i XOR j] := maximum of new_dp[i XOR j] and prev+cnt
dp := new_dp
return size of nums - new_dp[0]
Example
Let us see the following implementation to get better understanding
def solve(nums, k): LIMIT = 2**10 temp = [[0 for _ in range(LIMIT)] for _ in range(k)] for i,x in enumerate(nums): temp[i%k][x] += 1 dp = [-2000 for _ in range(LIMIT)] dp[0] = 0 for row in temp: maxprev = max(dp) new_dp = [maxprev for _ in range(LIMIT)] for i,cnt in enumerate(row): if cnt > 0: for j,prev in enumerate(dp): new_dp[i^j] = max(new_dp[i^j], prev+cnt) dp = new_dp return len(nums) - new_dp[0] nums = [3,4,5,2,1,7,3,4,7] k = 3 print(solve(nums, k))
Input
[3,4,5,2,1,7,3,4,7], 3
Output
-9