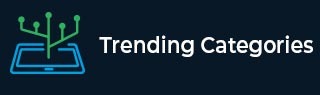
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find two pairs of numbers where difference between sum of these pairs are minimized in python
Suppose we have a list of numbers called nums and we want to select two pairs of numbers from it such that the absolute difference between the sum of these two pairs is minimized.
So, if the input is like nums = [3, 4, 5, 10, 7], then the output will be 1, as we can select these pairs (3 + 7) - (4 + 5) = 1.
To solve this, we will follow these steps:
- distances := a new list
- for i in range 0 to size of nums - 2, do
- for j in range i + 1 to size of nums - 1, do
- insert a list [|nums[i] - nums[j]| , i, j] at the end of distances
- sort the list distances
- ans := 1^9
- for i in range 0 to size of distances - 2, do
- [dist, i1, i2] := distances[i]
- j := i + 1
- [dist2, i3, i4] := distances[j]
- while j < size of distances and elements in (i1, i2, i3, i4) are not unique, do
- [dist2, i3, i4] := distances[j]
- j := j + 1
- if elements in (i1, i2, i3, i4) are unique, then
- ans := minimum of ans and (dist2 - dist)
- return ans
- for j in range i + 1 to size of nums - 1, do
Let us see the following implementation to get better understanding:
Example Code
class Solution: def solve(self, nums): distances = [] for i in range(len(nums) - 1): for j in range(i + 1, len(nums)): distances.append((abs(nums[i] - nums[j]), i, j)) distances.sort() ans = 1e9 for i in range(len(distances) - 1): dist, i1, i2 = distances[i] j = i + 1 dist2, i3, i4 = distances[j] while j < len(distances) and len({i1, i2, i3, i4}) != 4: dist2, i3, i4 = distances[j] j += 1 if len({i1, i2, i3, i4}) == 4: ans = min(ans, dist2 - dist) return ans ob = Solution() nums = [3, 4, 5, 10, 7] print(ob.solve(nums))
Input
[3, 4, 5, 10, 7]
Output
1
Advertisements