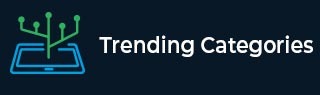
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find smallest difference of angles of two parts of a given circle in C++
In this article, we will find the smallest difference in angles between two parts of a given circle using the C++ programming language. Before proceeding to the code, let’s first understand how we can calculate the angles to find the smallest difference between them.
We are given the angles of all the pieces of the circle in the array. We have to join the pieces in such a way that the angle difference between the two pieces is the least.
Let's go through the input and output scenario for better understanding. Here, we declare an array contianing three different angles of a circle as shown below −
Input
ang[] = {160, 120, 80}
Output
40
Explanation
In this input and output scenario given the angles of the three pieces: 120°, 80°, and 160°, we want to join two of these pieces to form sectors with the smallest difference in angle.
Step 1: We can choose the 120° and 80° pieces. The sum of two angles is 120° and 80°, resulting in a total of (120 + 80 = 200).
Step 2: The difference between the combined angle and the remaining piece (160°) is: [ |(120° + 80°) - 160°| = |200° - 160°| = 40° ]
Following is the diagram of a circle where we draw an angles −

Solution Approach
Here, we have to merge all parts to make two parts. And for that, we need to take continuous parts (in the example we can't take ang1 and ang3 together).
Let's take the angle of part1 be A.
Then the angle of part2 will be 360 - A.
The difference will be |A - (360 - A)|. We have taken absolute value as there can only be positive angles.
Solving the difference equation,
2 * |A - 180|, this needs to be the minimum value. And for that, we will try to merge all possible parts of the circle and find the minimum value for (2*|A - 180|).
Example
Following is the program to find smallest difference of angles of two parts of a given circle in C++ −
#include <iostream> #include <math.h> using namespace std; int CalcSmallDiffAng(int ang[], int n) { int Left = 0, A = 0, minDiff = 360; for (int i = 0; i < n; i++) { A += ang[i]; while (A >= 180) { minDiff = min(minDiff, 2 * abs(180 - A)); A -= ang[Left]; Left++; } minDiff = min(minDiff, 2 * abs(180 - A)); } return minDiff; } int main() { int ang[] = { 160, 120, 80 }; int n = sizeof(ang) / sizeof(ang[0]); cout<<"The smallest difference of angles of two parts of a given circle is "<<CalcSmallDiffAng(ang, n); return 0; }
Output
The above program displays the following output −
The smallest difference of angles of two parts of a given circle is 40