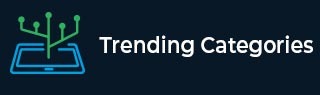
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find size of smallest sublist whose sum at least target in Python
Suppose we have a list of numbers called nums, and an another input called target, we have to find the size of the shortest sublist such that its sum value is same as target or larger. If there is no such sublist then return -1.
So, if the input is like nums = [2, 11, -4, 17, 4] target = 19, then the output will be 2, as we can select [17, 4] to get sum of at least 19.
To solve this, we will follow these steps −
ps := a list with only one element 0
for each num in nums, do
insert (last element of ps + num) after ps
if num >= target, then
return 1
min_size := inf
q := [0]
j := 0
for i in range 1 to size of ps, do
j := minimum of j, size of q - 1
while j < size of q and ps[i] - ps[q[j]] >= target, do
min_size := minimum of min_size and (i - q[j])
j := j + 1
while q and ps[i] <= ps[last element of q], do
delete last element from q
insert i at the end of q
return min_size if min_size < inf otherwise -1
Example
Let us see the following implementation to get better understanding −
class Solution: def solve(self, nums, target): ps = [0] for num in nums: ps += [ps[-1] + num] if num >= target: return 1 min_size = float("inf") q = [0] j = 0 for i in range(1, len(ps)): j = min(j, len(q) - 1) while j < len(q) and ps[i] - ps[q[j]] >= target: min_size = min(min_size, i - q[j]) j += 1 while q and ps[i] <= ps[q[-1]]: q.pop() q.append(i) return min_size if min_size < float("inf") else -1 ob = Solution() nums = [2, 11, -4, 17, 4] target = 19 print(ob.solve(nums, target))
Input
[2, 11, -4, 17, 4], 19
Output
2