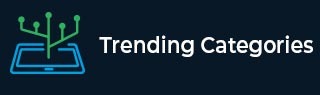
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find replicated list by replicating each element n times
Suppose we have a list of n elements; we have to repeat each element in the list n number of times.
So, if the input is like nums = [1,5,8,3], then the output will be [1, 1, 1, 1, 5, 5, 5, 5, 8, 8, 8, 8, 3, 3, 3, 3]
To solve this, we will follow these steps −
- n := size of nums
- ret := a new list
- for each num in nums, do
- ret := ret concatenate a list with n number of nums
- return ret
Example
Let us see the following implementation to get better understanding
def solve(nums): n = len(nums) ret = [] for num in nums: ret += [num]*n return ret nums = [1,5,8,3] print(solve(nums))
Input
[1,5,8,3]
Output
[1, 1, 1, 1, 5, 5, 5, 5, 8, 8, 8, 8, 3, 3, 3, 3]
Advertisements