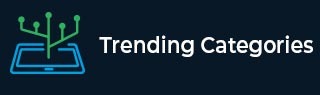
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find out the minimum moves in a snakes and ladders game in Python
Suppose we are playing a game of snakes and ladders. We have a condition that we can roll any number that we can like on a dice. We start from position 0 and our destination is position 100, and we roll the dice several times to reach the destination. We must find out the least number of dice rolls required to reach the destination if we are provided with the position of the snakes and ladders on the board.The arrays snakes and ladders represent the positions of snakes and ladders in the board and each entry in the arrays contains a start value and an end value of a snake or a ladder on the board.
So, if the input is like ladders = [(11, 40), (37,67),(47, 73),(15, 72)], snakes = [(90, 12), (98, 31), (85, 23), (75, 42), (70, 18), (49, 47)], then the output will be 8.
The minimum number of moves required is 8 to reach the 100th position on the board given the positions of snakes and ladders.
To solve this, we will follow these steps −
- append the array snakes to array ladders
- edges := a new map
- for each pair f,t in ladders, do
- edges[f] := t
- u := a new set
- v := a new set
- add(1) to v
- m := 0
- while 100 is not present in v, do
- m := m + 1
- w := a new set
- for each f in v, do
- for i in range 1 to 6, do
- n := f + i
- if n is present in edges, then
- n := edges[n]
- if n is present in u, then
- go for next iteration
- add(n) to u
- add(n) to w
- for i in range 1 to 6, do
- v := w
- return m
Example
Let us see the following implementation to get better understanding −
def solve(ladders, snakes): ladders.extend(snakes) edges = {} for f,t in ladders: edges[f] = t u = set() v = set() v.add(1) m = 0 while 100 not in v: m += 1 w = set() for f in v: for i in range(1,7): n = f + i if n in edges: n = edges[n] if n in u: continue u.add(n) w.add(n) v = w return m print(solve([(11, 40), (37,67),(47, 73),(15, 72)], [(90, 12), (98, 31), (85, 23), (75, 42), (70, 18), (49, 47)]))
Input
[(11, 40), (37,67),(47, 73),(15, 72)], [(90, 12), (98, 31), (85, 23), (75, 42), (70, 18), (49, 47)]
Output
8