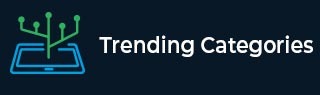
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find out the letter at a particular index in a synthesized string in python
Suppose, we are given a string 'input_str'. Now, we are asked to determine every possible substring from the given string then concatenate all the substrings one after another in a lexical order into another string. We are also provided an integer value k. Our task is to return the letter at index k from the concatenated string.
So, if the input is like input_str = 'pqrs', k = 6, then the output will be p
The substrings from the given string in lexical order are p, pq, pqr, pqrs, q, qr, qrs, r, rs, s.
If we concatenate the strings, it becomes ppqpqrpqrsqqrqrsrrss. At position 6, the letter is 'p'. (indexing starts at 0).
To solve this, we will follow these steps −
- stk_list := a new list containing a tuple that contains a blank string and a list of all the letters from input_str
- while stk_list is not empty, do
- pre := delete last element from stk_list
- temp := delete last element from stk_list
- if k < size of pre, then
- return pre[k]
- k := k - size of pre
- input_sorted := a new list that contains tuples that contain the letters of the input_str and their position in input_str
- sort the list input_sorted based on the second value of the tuples in a descending order
- i := 0
- while i < size of input_sorted, do
- val := input_sorted[i, 0]
- temp1 := [input_sorted[i, 1]]
- j := i + 1
- while j < size of input_sorted and input_sorted[j, 0] is same as val, do
- insert input_sorted[j, 1] at the end of temp1
- j := j + 1
- insert (pre+val, temp1) at the end of stk_list
- i := j
- return null
Example
Let us see the following implementation to get better understanding −
def solve(input_str, k): stk_list = [("",list(range(len(input_str))))] while stk_list: pre, temp = stk_list.pop() if k < len(pre): return pre[k] k -= len(pre) input_sorted = sorted([(input_str[i],i+1) for i in temp if i < len(input_str)], reverse=True) i = 0 while i < len(input_sorted): val = input_sorted[i][0] temp1 = [input_sorted[i][1]] j = i + 1 while j < len(input_sorted) and input_sorted[j][0]== val: temp1.append(input_sorted[j][1]) j += 1 stk_list.append((pre+val, temp1)) i = j return None print(solve('pqrs', 6))
Input
'pqrs', 6
Output
p
Advertisements