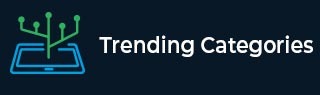
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to Find Out the Cost after Finding k Unique Subsequences From a Given String in C++
Suppose, we have a string s and another value k. We have to select some subsequences of s, so that we can get k unique subsequences. Here, the cost of selecting a subsequence equals the length of (s) - length of (subsequence). So, we have to find the lowest total cost possible after selecting k unique subsequences. If we are unable to find out this set, we will return -1. We will consider the empty string as a valid subsequence.
So, if the input is like s = "pqrs", k = 4, then the output will be 3.
To solve this, we will follow these steps −
n := size of s
Define one 2D array dp of size (n + 1) x (n + 1) and initialize it with 0
Define one map last
dp[0, 0] := 1
for initialize i := 0, when i < n, update (increase i by 1), do −
dp[i + 1, 0] := 1
for initialize j := (i + 1), when j >= 1, update (decrease j by 1), do −
dp[i + 1, j] := dp[i, j] + dp[i, j - 1]
if s[i] is not the end element of last, then −
for initialize j := 0, when j <= last[s[i]], update (increase j by 1), do −
dp[i + 1, j + 1] - = dp[last[s[i]], j]
last[s[i]] := i
cost := 0
for initialize i := n, when i >= 0, update (decrease i by 1), do −
val := minimum of k and dp[n, i]
cost := cost + (val * (n - i))
k := k - dp[n, i]
if k <= 0, then −
Come out from the loop
if k <= 0, then −
return cost
return -1
Example
Let us see the following implementation to get better understanding −
#include <bits/stdc++.h> using namespace std; int solve(string s, int k) { int n = s.size(); vector<vector<int>> dp(n + 1, vector<int>(n + 1, 0)); unordered_map<char, int> last; dp[0][0] = 1; for (int i = 0; i < n; i++) { dp[i + 1][0] = 1; for (int j = (i + 1); j >= 1; j--) { dp[i + 1][j] = dp[i][j] + dp[i][j - 1]; } if (last.find(s[i]) != last.end()) { for (int j = 0; j <= last[s[i]]; j++) { dp[i + 1][j + 1] -= dp[last[s[i]]][j]; } } last[s[i]] = i; } int cost = 0; for (int i = n; i >= 0; i--) { int val = min(k, dp[n][i]); cost += (val * (n - i)); k -= dp[n][i]; if (k <= 0) { break; } } if (k <= 0) { return cost; } return -1; } int main(){ cout << solve("pqrs",4) << endl; return 0; }
Input:
"pqrs", 4
Output
3