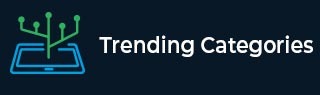
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find number of moves to win deleting repeated integer game in Python
Suppose two friends Amal and Bimal are playing a game with a sorted list of numbers called nums. In this game in a single turn, Amal chooses any three numbers. Bimal removes one of them, and then Amal removes one of them. The list starts out with an odd number of elements. Here Amla wishes to minimize the number of turns required to make the list contain no repeated elements, Bimal wishes to maximize the number of turns. If Amal and Bimal act optimally, we have to find how many turns are needed for this game.
So, if the input is like nums = [1, 1, 2, 3, 3, 3, 4], then the output will be 2, as If Amal selects [1, 1, 3], then Bimal removes 3 to maximize turns, the array is [1, 1, 2, 3, 3, 4], Amal removes 1, so array is [1,2,3,3,4], then in the next turn Amal selects [3,3,4], then Bimal will remove 4 to maximize turns. So then Amal can remove 3 and array will be [1,2,3], there is no duplicate elements.
To solve this, we will follow these steps −
repeats := 0
for i in range 1 to size of nums, do
if nums[i] is same as nums[i-1], then
repeats := repeats + 1
return quotient of (repeats + 1) / 2
Let us see the following implementation to get better understanding −
Example
class Solution: def solve(self, nums): repeats = 0 for i in range(1, len(nums)): if nums[i] == nums[i-1]: repeats += 1 return (repeats + 1) // 2 ob = Solution() nums = [1, 1, 2, 3, 3, 3, 4] print(ob.solve(nums))
Input
[1, 1, 2, 3, 3, 3, 4]
Output
2