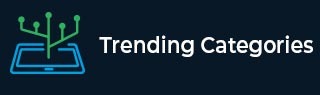
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find number of combinations of coins to reach target in Python
Suppose we have a list of coins and another value amount, we have to find the number of combinations there are that sum to amount. If the answer is very large, then mod the result by 10^9 + 7.
So, if the input is like coins = [2, 5] amount = 10, then the output will be 2, as we can make these combinations − [2, 2, 2, 2, 2], [5, 5]
To solve this, we will follow these steps −
- m := 10^9 + 7
- dp := a list of size same as amount + 1, and fill it with 0
- dp[0] := 1
- for each d in coins, do
- for i in range 1 to size of dp, do
- if i - d >= 0, then
- dp[i] := dp[i] + dp[i - d]
- if i - d >= 0, then
- for i in range 1 to size of dp, do
- return (last element of dp) mod m
Let us see the following implementation to get better understanding −
Example
class Solution: def solve(self, coins, amount): dp = [0] * (amount + 1) dp[0] = 1 for d in coins: for i in range(1, len(dp)): if i - d >= 0: dp[i] += dp[i - d] return dp[-1] % (10 ** 9 + 7) ob = Solution() coins = [2, 5] amount = 10 print(ob.solve(coins, amount))
Input
[2, 5], 10
Output
2
Advertisements