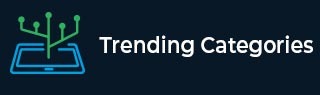
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find nth Fibonacci term in Python
Suppose we have a number n. We have to find the nth Fibonacci term by defining a recursive function.
So, if the input is like n = 8, then the output will be 13 as first few Fibonacci terms are 0, 1, 1, 2, 3, 5, 8, 13, 21, 34...
To solve this, we will follow these steps −
- Define a function solve() . This will take n
- if n <= 2, then
- return n - 1
- otherwise,
- return solve(n - 1) + solve(n - 2)
Example
Let us see the following implementation to get better understanding −
def solve(n): if n <= 2: return n - 1 else: return solve(n - 1) + solve(n - 2) n = 8 print(solve(n))
Input
8
Output
13
Advertisements