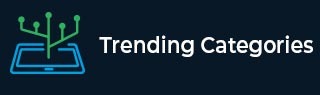
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find nearest number of n where all digits are odd in python
Suppose we have a number n, we have to find the next closest value where all digits are odd. When there are two values tied for being closest to n, return the larger one.
So, if the input is like n = 243, then the output will be 199.
To solve this, we will follow these steps −
- first_even := -1
- s := n as string
- l := size of s
- for i in range 0 to l, do
- if s[i] is even, then
- first_even := i
- come out from the loop
- if s[i] is even, then
- if first_even is same as -1, then
- return n
- big := 1 + numeric value of s[from index 0 to i]
- if s[i] is same as "0", then
- if s[i - 1] is same as "1", then
- small := numeric value of s[from index 0 to i] - 1
- otherwise,
- small := numeric value of s[from index 0 to i] - 11
- if s[i - 1] is same as "1", then
- otherwise,
- small := numeric value of s[from index 0 to i] - 1
- for i in range i + 1 to l, do
- big := big concatenate "1"
- small := small concatenate "9"
- big := numeric value of big, small := numeric value of small
- d2 := big - n, d1 := n - small
- if d1 < d2, then
- return small
- otherwise when d1 >= d2, then
- return big
Let us see the following implementation to get better understanding −
Example
class Solution: def solve(self, n): first_even = -1 s = str(n) l = len(s) for i in range(l): if int(s[i]) % 2 == 0: first_even = i break if first_even == -1: return n big = str(int(s[: i + 1]) + 1) if s[i] == "0": if s[i - 1] == "1": small = str(int(s[: i + 1]) - 1) else: small = str(int(s[i : i + 1]) - 11) else: small = str(int(s[: i + 1]) - 1) for i in range(i + 1, l): big += "1" small += "9" big, small = int(big), int(small) d2 = big - n d1 = n - small if d1 < d2: return small elif d1 >= d2: return big ob = Solution() n = 243 print(ob.solve(n))
Input
243
Output
199
Advertisements